In our series of JavaScript programs, we are going to learn how to find armstrong number in JS using various methods. For aspiring web developers, it is a crucial exercise to lay the foundation of programming with JavaScript.
The JavaScript program for armstrong number helps you develop logical thinking and problem-solving skills that are the bread and butter of successful coding.
But first, let’s understand what exactly is an armstrong number.
An Armstrong number is a number that equals the sum of its own digits each raised to the power of the number of digits.
Example:
Take, for example, the number 153. It’s a 3-digit number, and if we take each digit, raise it to the power of 3, and sum them up (13 + 53 + 33), the result is 153 itself.
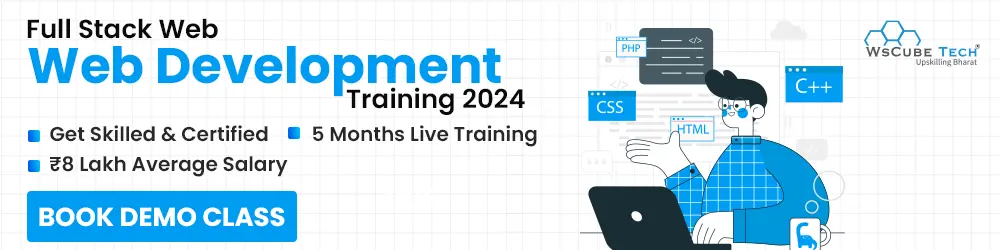
This self-representative property makes 153 an Armstrong number, a concept that not only tickles the mathematicians but also serves as an excellent exercise in programming.
In this post, we’ll guide you through different ways to program the solution to finding Armstrong numbers in JavaScript. Each method is designed to introduce you to fundamental programming concepts in JavaScript, from loops and conditionals to functions and ES6 features.
And if you’re serious about taking your skills to the next level, consider enrolling in the online full-stack developer course by WsCube Tech.
Also learn: How to Print Multiplication Table in JavaScript? (using for, while, recursion, html)
Armstrong Number in JavaScript Using for Loop
Below is a armstrong number program in JavaScript using for loop, and shows output based on user input:
JavaScript Code:
const readline = require('readline').createInterface({
input: process.stdin,
output: process.stdout
});
readline.question('Enter a number: ', number => {
if (isArmstrongNumber(number)) {
console.log(`${number} is an Armstrong Number.`);
} else {
console.log(`${number} is not an Armstrong Number.`);
}
readline.close();
});
function isArmstrongNumber(number) {
let sum = 0;
const numberOfDigits = number.length;
for (let digit of number) {
sum += Math.pow(parseInt(digit), numberOfDigits);
}
return sum === parseInt(number);
}
Output:
Enter a number: 371
371 is an Armstrong Number.
Explanation:
- The readline module creates an interface for reading data from the console. It prompts the user to enter a number.
- The question method is used to display a message to the user, asking them to enter a number. Once the user enters a number and presses enter, the provided callback function is executed.
- The input number is passed to the isArmstrongNumber function, which checks if it is an Armstrong number. This function works iterates through each digit of the input number.
- Based on the return value of isArmstrongNumber, it logs to the console whether the input number is an Armstrong number.
- Finally, the readline interface is closed with readline.close().
Upskill Yourself With Live Training
Armstrong Number Program in JavaScript Using while Loop
To create a program that checks for Armstrong numbers using a while loop, you can modify the previous example to incorporate a while loop for iterating through the digits of the number.
JavaScript Code:
const readline = require('readline').createInterface({
input: process.stdin,
output: process.stdout
});
readline.question('Enter a number: ', number => {
if (isArmstrongNumber(number)) {
console.log(`${number} is an Armstrong Number.`);
} else {
console.log(`${number} is not an Armstrong Number.`)
}
readline.close();
});
function isArmstrongNumber(number) {
let sum = 0;
const numberOfDigits = number.length;
let tempNumber = parseInt(number);
while (tempNumber > 0) {
let digit = tempNumber % 10;
sum += Math.pow(digit, numberOfDigits);
tempNumber = Math.floor(tempNumber / 10); // Move to the next digit
}
return sum === parseInt(number);
}
Output:
Enter a number: 407
407 is an Armstrong Number.
Explanation:
- Using the readline module, the program prompts the user to enter a number.
- isArmstrongNumber function is designed to check if the provided number is an Armstrong number.
- It initially converts the input number (string) to an integer and calculates the number of digits.
- A while loop is used to iterate over each digit of the number.
Within the loop:
- The last digit of tempNumber is extracted using the modulo operation (tempNumber % 10).
- This digit is then raised to the power of numberOfDigits and added to sum.
- tempNumber is divided by 10 (and floored to an integer) to remove the last digit, moving to the next digit in the next iteration.
- After calculating the sum of the digits each raised to the power of the number of digits, it compares the sum to the original number. If they are equal, it indicates the number is an Armstrong number.
- The result is printed to the console, indicating whether the entered number is an Armstrong number.
- Finally, the readline interface is closed.
Also read: Find Factorial in JavaScript (3 Programs & Code)
Armstrong Number in JS Using toString and split Methods
To check if a given number is an Armstrong number in JavaScript, you can use the toString and split methods to work with each digit of the number individually.
JavaScript Code:
function isArmstrongNumber(number) {
// Convert the number to a string and then split it into an array of digits
const digits = number.toString().split('');
const numberOfDigits = digits.length;
// Use Array.prototype.reduce() to calculate the sum of the digits each raised to the power of numberOfDigits
const sum = digits.reduce((accumulator, digit) => {
return accumulator + Math.pow(parseInt(digit, 10), numberOfDigits);
}, 0);
// Check if the sum is equal to the original number
if (sum === number) {
console.log(`${number} is an Armstrong Number.`);
} else {
console.log(`${number} is not an Armstrong Number.`);
}
}
// Example usage
isArmstrongNumber(153);
isArmstrongNumber(123);
Output:
153 is an Armstrong Number.
123 is not an Armstrong Number.
Explanation:
- The number is first converted into a string to access the split method, which splits the string into an array of single-character strings, each representing a digit of the original number.
- The reduce method is used to iterate over the array of digits. For each digit, it converts the digit back into an integer (using parseInt(digit, 10)) and then raises it to the power of the total number of digits (the length of the array). The result is accumulated to produce the sum of all digits each raised to the power of the number of digits.
- Finally, the function checks if the calculated sum is equal to the original number. If they are equal, the function logs to the console that the number is an Armstrong number. Otherwise, it logs that it is not.
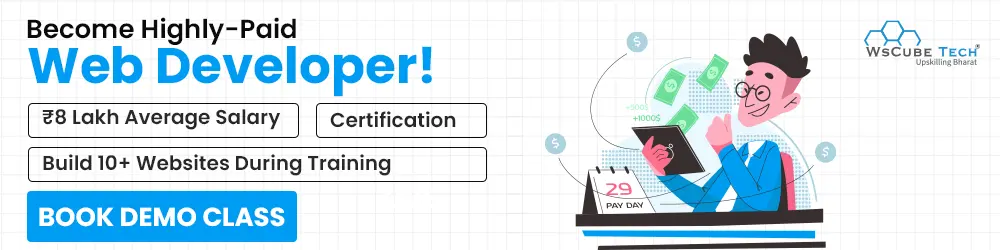
Armstrong Number in JS Using Array.from Method
To create a JavaScript function that checks for Armstrong numbers using the Array.from method, you can leverage the method to directly create an array from the number’s string representation. This approach is elegant and concise, making use of modern JavaScript features for clean and readable code.
JavaScript Code:
function isArmstrong(number) {
// Create an array of digits from the number
const digits = Array.from(number.toString(), Number);
const order = digits.length;
const sum = digits.reduce((acc, digit) => acc + Math.pow(digit, order), 0);
if (sum === number) {
console.log(`${number} is an Armstrong Number`);
} else {
console.log(`${number} is not an Armstrong Number`);
}
}
// Example calls
isArmstrong(9474);
isArmstrong(520);
Output:
9474 is an Armstrong Number
520 is not an Armstrong Number
Explanation:
Array.from(number.toString(), Number) converts the number to a string, and then Array.from creates an array where each element is a digit of the original number. The second argument to Array.from, Number, is a map function that converts each string digit back into a number.
The length of the digits array (order) determines how many digits the number has, which is used as the exponent when calculating the sum of each digit raised to the power of the number of digits.
The reduce method accumulates the sum of each digit raised to the order power. It starts with an accumulator (acc) initialized to 0 and iterates through each digit, adding Math.pow(digit, order) to the accumulator.
If the calculated sum is equal to the original number, it means the number is an Armstrong number, and this result is logged to the console. Otherwise, it logs that the number is not an Armstrong number.
Interview Questions for You to Prepare for Jobs
Armstrong Number in JS Using Array Reduce Method
Here, we will learn how to find armstrong number in JavaScript using the array reduce method. This method aligns well with functional programming principles and allows for concise, readable code.
JavaScript Code:
function isArmstrong(number) {
// Convert the number to an array of its digits
const digits = number.toString().split('').map(Number);
const order = digits.length;
// Calculate the sum of the digits each raised to the power of the number of digits
const sum = digits.reduce((accumulator, digit) => accumulator + Math.pow(digit, order), 0);
// Check if the sum equals the original number
if (sum === number) {
console.log(`${number} is an Armstrong Number.`);
} else {
console.log(`${number} is not an Armstrong Number.`);
}
}
// Example calls
isArmstrong(153);
isArmstrong(123);
Output:
153 is an Armstrong Number.
123 is not an Armstrong Number.
Explanation:
The number is first converted to a string and then split into an array of single-character strings. Each string is then converted back to a number using the map(Number) function, resulting in an array of digits.
The length property of the digits array gives the order (number of digits) of the original number, which is used as the exponent in the sum calculation.
The reduce method iterates over each digit in the array, calculating the sum of the digits each raised to the power of the order. The reduce method takes two parameters: an accumulator (initially set to 0) and the current digit. For each digit, it adds the digit raised to the power of the order to the accumulator.
Finally, the function checks if the sum calculated is equal to the original number. If they match, it means the number is an Armstrong number, and a message is logged to the console. Otherwise, a message indicating the number is not an Armstrong number is logged.
Also read: Print Fibonacci Series in JavaScript (6 Programs With Code)
Role of JavaScript Armstrong Number Program
1. Understanding Basic Programming Constructs
The Armstrong Number Program in JavaScript introduces beginners to fundamental programming concepts such as loops (for, while), conditional statements (if-else), and basic arithmetic operations.
JavaScript, being a versatile and widely-used web development language, provides a practical context for applying these constructs.
2. Familiarity with JavaScript Syntax and Best Practices
Through the process of writing a program to check Armstrong number in JavaScript, you get hands-on experience with JS syntax. This includes defining variables, using operators, and writing functions. Additionally, you get to explore best practices in coding such as commenting, naming conventions, and code structuring for readability and maintainability.
3. Problem-Solving and Logical Thinking
The Armstrong number problem is essentially a puzzle that requires a logical approach to solve. It challenges you to think about how to break down the problem into smaller steps, apply mathematical concepts, and translate these steps into JavaScript code. This kind of problem-solving is crucial in all areas of software development.
4. Debugging and Testing Skills
Debugging is an essential skill for any developer. Writing a program to find Armstrong numbers in JS provides an opportunity to practice debugging techniques as you test your code for correctness and handle potential errors. You learn to use console logs, breakpoints, and other debugging tools available in JavaScript environments.
5. Introduction to Mathematical Concepts in Programming
The JavaScript Armstrong Number Program is a great way to illustrate how mathematical concepts are applied in programming. It requires understanding how to work with numbers, powers, and sums—skills that are relevant in many programming tasks, from algorithms and data processing to game development and simulations.
6. Enhancing Creativity and Experimentation
Once the basic program is in place, you can enhance it, such as by finding all Armstrong numbers within a range or optimizing their solution for efficiency. This fosters creativity and encourages experimentation with different approaches, which is a key aspect of becoming an effective developer.
7. Preparation for More Complex Programming Challenges
Starting with simpler programming exercises like the JavaScript Armstrong number program prepares you for tackling more complex challenges. As you grow more confident in your ability to solve problems with JS, you can move on to more sophisticated projects that involve interacting with web APIs, manipulating the DOM, or using frameworks and libraries.
Also read: Check Prime Number in JavaScript (5 Programs)
Common Mistakes to Avoid While Writing JavaScript Programs
While writing JavaScript programs, beginners often encounter common pitfalls that can lead to errors or inefficient code. Being aware of these mistakes helps avoid them and improve coding skills.
1. Not Using let or const to Declare Variables
Using var to declare variables or not using any keyword at all can lead to unexpected behavior due to var’s function scope and hoisting. Always use let for variables that change and const for variables that remain constant.
2. Forgetting About Scope
JavaScript has function scope and block scope. Not understanding these scopes can lead to unexpected behavior in your code. Ensure you understand how scoping works, especially when using let, const, and var.
3. Misunderstanding this Keyword
The value of this can be confusing, as it changes based on the context in which a function is called. Arrow functions and regular functions handle this differently, so be cautious and understand the context in which you’re using it.
4. Ignoring Semicolons
While JavaScript has automatic semicolon insertion (ASI), relying on it can lead to hard-to-debug errors. It’s best practice to explicitly use semicolons to mark the end of statements.
5. Mutable Data Confusion
Not understanding the difference between primitive values (which are immutable) and objects (which are mutable) can lead to bugs. For instance, when you assign an object to another variable, you’re copying the reference, not the value.
6. Overlooking Hoisting
Variables and function declarations are hoisted to the top of their scope, not their assignments. This can lead to errors if you try to use a variable before it is declared and assigned.
7. Not Using Strict Mode
Strict mode (“use strict”;) can help catch common coding mistakes and unsafe actions (like accessing global objects). It’s a good practice to start your scripts with strict mode.
8. Misusing Equality Operators
JavaScript has both strict (===, !==) and type-converting (==, !=) equality comparisons. Using the type-converting versions without understanding type coercion can lead to unexpected results.
9. Forgetting to Return Values
When you create a function that’s supposed to return a value, forgetting the return statement will cause the function to return undefined instead.
10. Callbacks and Asynchronous Code Mismanagement
JavaScript is heavily asynchronous, especially in environments like Node.js or when dealing with AJAX requests in the browser. Not properly managing asynchronous code can lead to callbacks that never execute or execute in the wrong order. Promises and async/await syntax can help manage asynchronous operations more cleanly.
11. Not Handling Errors
Failing to catch and handle errors in your code can lead to unresponsive applications and poor user experience. Always use try-catch blocks or promise rejection handlers when dealing with operations that might fail.
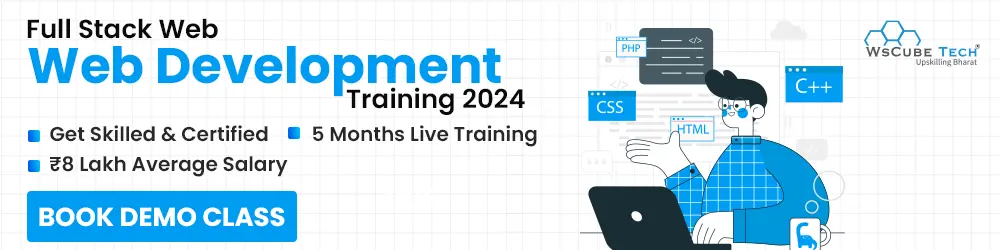
FAQs About JavaScript Armstrong Number
An Armstrong number, also known as a narcissistic number, is a number that is equal to the sum of its own digits each raised to the power of the number of digits.
This exercise helps beginners understand and practice fundamental programming concepts such as loops, conditionals, and mathematical operations in JavaScript. It’s a practical way to apply basic JavaScript syntax and logic to solve problems.
Yes, Armstrong numbers can have any number of digits. However, the more digits they have, the rarer they become. The exercise of finding Armstrong numbers is not just about the calculation but also understanding the logic and limitations of what you are trying to achieve.
JavaScript is a versatile and widely-used programming language that can run both in a web browser and on a server (using Node.js). Learning to write programs like the Armstrong number checker in JavaScript can be a stepping stone to web development and understanding more complex programming concepts.
Technically, you can search for Armstrong numbers in any range, but practical limits come from the computing resources (such as time and memory) available. In exercises, ranges are usually small enough to be computationally feasible on any modern computer.
Optimization can involve reducing the number of calculations (for example, by recognizing that no four-digit number can be an Armstrong number) or improving the algorithm’s efficiency (such as by minimizing the number of times you convert between numbers and strings or iterating through digits).
In JavaScript, == is the equality operator that performs type coercion if the operands are of different types, while === is the strict equality operator that does not perform type coercion. For checking Armstrong numbers, it’s best to use === to ensure that the types (number) are also considered in the comparison, avoiding unexpected type coercion.
Absolutely! Using ES6 features like arrow functions, const and let for variable declarations, template literals, and the Array.from method can make your Armstrong number program more concise and readable. It’s also a good practice to familiarize yourself with modern JavaScript syntax and features.
Start by checking for common syntax errors or typos. Use console.log statements to print out intermediate values and ensure your logic is correct at each step. Additionally, consider using a debugger tool to step through your code line by line and inspect variables.
Free Courses for You
Wrapping Up:
Learning the JavaScript program for armstrong numbers shows the beauty of programming and mathematical logic, serving as an excellent primer for beginners. However, this is just the tip of the iceberg.
To truly use the power of JavaScript and level-up your web development skills, consider enrolling in the live online JavaScript course or the best full-stack developer course. These courses offer structured learning paths, real-world projects, and live expert guidance, making them invaluable for upskilling and career building.