In our series of JavaScript programs, today, we will learn how to print multiplication table in JS.
At first glance, creating a multiplication table in JavaScript might seem like a simple task, yet it consolidates the essence of programming—breaking down a problem into manageable steps and using code to solve it.
This task will not only introduce you to basic programming concepts but also provide a hands-on opportunity to engage with JavaScript, a cornerstone language of the web.
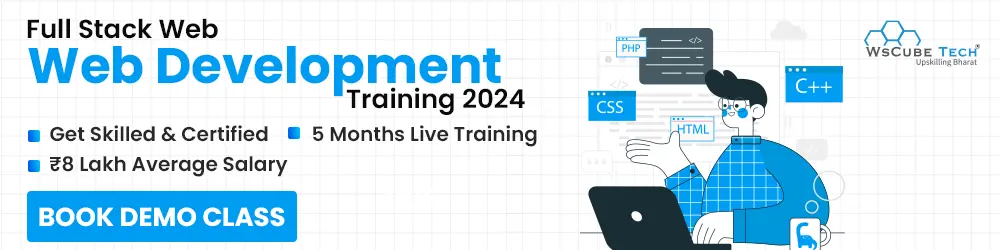
Understanding how to create multiplication table in JavaScript is more than just learning how to loop through numbers. It’s about grasping the core of programming logic and the skills essential for any aspiring web developer.
For those looking to build a high-paying career in web development, book the live demo class of online full stack developer course by WsCube Tech.
This training program not only cover the breadth of front-end and back-end technologies but also provide a structured curriculum to guide you from the basics to the advanced concepts of web development, including, of course, JavaScript.
Upskill Yourself With Live Training
Recommended Courses for You | Book Live Class for FREE! |
Full Stack Web Development Course | Book Now |
WordPress Course | Book Now |
Front-End Development Course | Book Now |
Multiplication Table in JavaScript Using for Loop
Below is a simple example of how to generate a multiplication table in JS using a for loop.
Code:
// Specify the number to generate the multiplication table for
const number = 5;
// Use a for loop to iterate from 1 to 10
for (let i = 1; i <= 10; i++) {
// Calculate the result of the current number times i
const result = number * i;
// Display the multiplication table in the console
console.log(`${number} * ${i} = ${result}`);
}
Output:
5 * 1 = 5
5 * 2 = 10
5 * 3 = 15
5 * 4 = 20
5 * 5 = 25
5 * 6 = 30
5 * 7 = 35
5 * 8 = 40
5 * 9 = 45
5 * 10 = 50
Explanation:
- The code starts by defining a constant number which holds the value for which we want to generate the multiplication table, in this case, 5.
- The for loop is set up with an iterator variable i starting at 1, the condition to continue the loop while i is less than or equal to 10, and an increment operation i++ to move to the next number in the table.
- Inside the loop, the code calculates the product of the current number (number) and the iterator (i). This result is stored in the result variable. It then uses console.log to print out the current step of the multiplication table in a readable format.
- For each iteration of the loop, i is increased by 1, and the loop continues until i exceeds 10, at which point the loop terminates.
This simple program shows the basics of using loops in JavaScript to perform repetitive tasks, such as generating a multiplication table. It’s a foundational exercise that helps beginners understand loop mechanics, variable manipulation, and outputting results, which are essential skills in programming.
Also learn: Check Prime Number in JavaScript (5 Programs)
Multiplication Table in JavaScript Using Recursion
Creating a multiplication table in JS using recursion is a more advanced concept compared to using a for loop. Recursion involves a function calling itself until it reaches a base condition that stops further calls.
Code:
function printMultiplicationTable(number, multiplier = 1) {
if (multiplier > 10) {
return; // Base condition to stop the recursion
}
console.log(`${number} * ${multiplier} = ${number * multiplier}`);
printMultiplicationTable(number, multiplier + 1); // Recursive call
}
// Generate and print the multiplication table for 5
printMultiplicationTable(5);
Output:
5 * 1 = 5
5 * 2 = 10
5 * 3 = 15
5 * 4 = 20
5 * 5 = 25
5 * 6 = 30
5 * 7 = 35
5 * 8 = 40
5 * 9 = 45
5 * 10 = 50
Explanation:
- The printMultiplicationTable function is defined with two parameters: number (the number to generate the multiplication table for) and multiplier (which tracks the current step in the multiplication table). The multiplier is initialized with a default value of 1.
- The recursion’s base condition is if (multiplier > 10), meaning the function will stop calling itself once the multiplier exceeds 10. This prevents infinite recursion and ensures the function eventually terminates.
- Within the function, the current multiplication result is calculated and printed using console.log. Then, the function calls itself (printMultiplicationTable(number, multiplier + 1)) with the multiplier incremented by 1. This recursive call repeats the process with the next step in the multiplication table.
- The function keeps calling itself with an incremented multiplier until the base condition is met, at which point the recursion stops.
Also learn: Print Fibonacci Series in JavaScript (6 Programs With Code)
Multiplication Table in JavaScript Using while loop
Now, let’s learn how to print a multiplication table in JavaScript using a while loop. This approach offers a slightly different mechanism compared to the for loop for iterating through numbers. The while loop continues to execute a block of code as long as a specified condition is true.
Code:
let i = 1; // Initialize the counter outside the loop
const number = 2; // Number to generate the multiplication table for
while (i <= 10) { // Condition to continue the loop
console.log(`${number} * ${i} = ${number * i}`);
i++; // Increment the counter
}
Output:
2 * 1 = 2
2 * 2 = 4
2 * 3 = 6
2 * 4 = 8
2 * 5 = 10
2 * 6 = 12
2 * 7 = 14
2 * 8 = 16
2 * 9 = 18
2 * 10 = 20
Explanation:
- Before entering the loop, the iterator i is initialized to 1, and the number variable is set to 2, representing the base number for the multiplication table.
- The while loop begins with a condition (i <= 10). As long as this condition evaluates to true, the loop will continue to execute. This ensures that the loop will run for the numbers 1 through 10.
- Inside the loop, the multiplication is performed, and the result is output to the console using console.log. The string template literal (${expression}) is used to insert the variables and expressions into the string for easy readability.
- At the end of each loop iteration, i is incremented by 1 (i++). This modification of the loop counter is crucial; without it, the loop would execute indefinitely, leading to an infinite loop.
- Once i exceeds 10, the condition (i <= 10) evaluates to false, and the loop terminates.
Interview Questions for You to Prepare for Jobs
Multiplication Table in HTML Using JavaScript
Creating a multiplication table in HTML using JavaScript includes dynamically generating the table elements in the HTML document. Below is a step-by-step guide on how to achieve this:
HTML Structure
First, set up the HTML structure to display the multiplication table. You’ll need an HTML table element where the table rows and cells will be added dynamically using JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Multiplication Table</title>
</head>
<body>
<h1>Multiplication Table</h1>
<table id="multiplicationTable">
<!-- Table content will be generated dynamically -->
</table>
<script src="multiplicationTable.js"></script>
</body>
</html>
JavaScript (multiplicationTable.js)
Now, create a JavaScript file to generate and populate the multiplication table within the <table> element. In this example, we’ll generate a multiplication table for numbers 1 to 10 for the base number 5.
// Get a reference to the table element
const table = document.getElementById('multiplicationTable');
// Define the number for which to generate the multiplication table
const baseNumber = 5;
// Generate the multiplication table
for (let i = 1; i <= 10; i++) {
// Create a table row
const row = document.createElement('tr');
// Create and populate table cells
const multiplierCell = document.createElement('td');
const resultCell = document.createElement('td');
multiplierCell.textContent = `${baseNumber} x ${i}`;
resultCell.textContent = baseNumber * i;
// Append cells to the row
row.appendChild(multiplierCell);
row.appendChild(resultCell);
// Append the row to the table
table.appendChild(row);
}
Explanation:
- We start by getting a reference to the HTML table element with the id “multiplicationTable.”
- Then, we define the base number for which we want to generate the multiplication table (in this case, 5).
- We use a for loop to iterate from 1 to 10, creating a new table row for each iteration.
- Inside each row, we create two table cells: one for the multiplication expression (e.g., “5 x 1”) and one for the result (e.g., “5”).
- We populate the cell contents accordingly.
- Finally, we append the row to the table.
Output:
5 x 1 5
5 x 2 10
5 x 3 15
5 x 4 20
5 x 5 25
5 x 6 30
5 x 7 35
5 x 8 40
5 x 9 45
5 x 10 50
This code dynamically generates the multiplication table using JavaScript and adds it to the HTML page, making it a dynamic and interactive part of your web content.
Also learn: Find Factorial in JavaScript (3 Programs & Code)
Role of Using JavaScript for Multiplication Table
Let’s know about the roles and benefits of JavaScript multiplication table program, especially to beginners in web development:
1. Understanding Programming Logic and Syntax
- Logic Development: It helps you understand the logic behind loops and conditions, which are fundamental in almost all programming tasks.
- Syntax Familiarity: JavaScript syntax is the backbone of web development. Through this program, you get familiar with basic syntax, variables, and operations.
2. Learning Control Structures
- Looping Mechanisms: Loops are crucial for iterating over data. The program to display multiplication table in JS introduces for loops, and while and do-while loops, teaching how to control flow within a program.
- Conditional Statements: Adding conditions to display certain multiplication tables introduces if statements, enhancing decision-making in code.
3. Function Use and Reusability
- Encapsulation: By wrapping the logic inside a function, you can understand the concept of encapsulation, making code modular and reusable.
- Parameters and Arguments: Passing the number for which the table is needed as a parameter to a function teaches about function arguments and parameter handling.
4. Debugging Skills
As a beginner, writing and debuging JS multiplication table programs, you will sharpen your problem-solving and debugging skills, crucial for any developer.
5. Foundational Step Towards Advanced Topics
Mastering the basics through JavaScript programs paves the way for learning more complex topics in JavaScript and web development, like asynchronous programming, API interactions, and framework utilization.
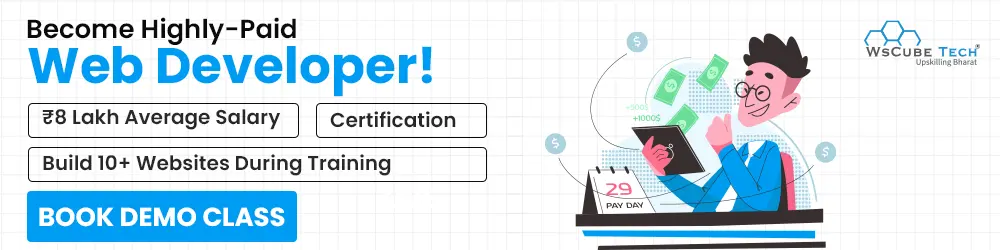
FAQs About JavaScript Multiplication Table
Learning to generate a multiplication table in JavaScript is important in web development because it serves as a foundational exercise to grasp key programming concepts. It helps beginners understand control structures like loops, basic syntax, and the manipulation of the Document Object Model (DOM). These skills are fundamental for creating dynamic and interactive websites, making it an essential starting point for anyone pursuing web development.
Yes, you can use other programming languages to create a multiplication table. However, JavaScript is particularly relevant in web development due to its ability to manipulate web page content. While other languages can be used for general calculations, JavaScript offers the advantage of seamlessly integrating with web technologies, allowing you to display the multiplication table on a webpage.
Both ‘for’ and ‘while’ loops can be used to generate a multiplication table, but they differ in syntax and usage. A ‘for’ loop is commonly used when you know the exact number of iterations, making it suitable for tasks like generating a fixed number of lines in a multiplication table.
On the other hand, a ‘while’ loop is more flexible and is used when the number of iterations is not known in advance or when the loop needs to continue until a specific condition is met.
You can make your multiplication table program interactive by incorporating user input. Use JavaScript’s prompt() or HTML form elements to gather input from users, allowing them to specify which number’s multiplication table they want to generate. Additionally, you can add buttons or event listeners to trigger the table generation, enhancing the user experience.
Free Courses for You
Course Name | Course Name |
Django Course | Affiliate Marketing Course |
Semrush Course | Video Editing Course |
Blogging Course | AngularJS Course |
Shopify Course | Photoshop Course |
Wrapping Up:
Learning how to print a multiplication table in JavaScript is not just about numbers—it’s about upskilling in web development. These skills are the building blocks of a successful developer journey.
To further enhance your expertise, book the live demo class of online JavaScript course or the online full-stack developer course by WsCube Tech. These courses offer hands-on experience, expert guidance, and a structured path to build your successful career in web development.
Happy coding!
Learn more JS programs