JavaScript language is known as the language of the web for its versatile capabilities. One of the basic yet powerful aspects of this language is generating random numbers in JavaScript.
This looks simple, but plays an important role in various ways. For example, in online games, random numbers are used to determine the outcome of dice rolls or card shuffles. This is one easy example. Like this, there are various uses of JavaScript random number generator program.
Hence, let’s learn how to generate a random number in JS, whether it’s between 0 and 1, 1 and 10, 1 and 100, any given range, or any-digit number.
Role of JavaScript Random Number Program
The JavaScript program to generate random numbers plays a role in various aspects of web development and programming, including:
- Enhancing User Experience in Web Apps: We can use random numbers to create dynamic and interactive user experiences. For example, online games use random numbers to generate the outcome of dice rolls or card shuffles, adding an element of unpredictability and excitement.
- Testing and Debugging: Developers often use random numbers to test the robustness of their applications. For instance, generating random inputs can help stress test or find unexpected bugs.
- Security Applications: In web security, random numbers are crucial, especially in cryptography. We can generate random secure tokens, passwords, and cryptographic keys, ensuring that these elements are difficult to predict or replicate.
- Data Analysis and Simulation: Random numbers are important in simulations and modeling real-world phenomena in areas like finance, science, or engineering. They help in creating diverse scenarios for predictive analysis.
- Load Balancing and Resource Allocation: In web services and cloud computing, random numbers can be used for distributing tasks or resources among multiple servers or processes in a balanced manner.
- Creative Web Design: Random numbers can bring an element of surprise and creativity in web design, like randomizing backgrounds, animations, or layout elements for a unique user experience each time a page is loaded.
- Algorithm Implementation: Many algorithms, especially in machine learning and AI, rely on random numbers for processes like random initialization, stochastic optimization, or in algorithms like Monte Carlo simulations.
- Ad and Content Display: Random numbers can decide which ads or content variations to show to different users, making online advertising and content delivery more dynamic and personalized.
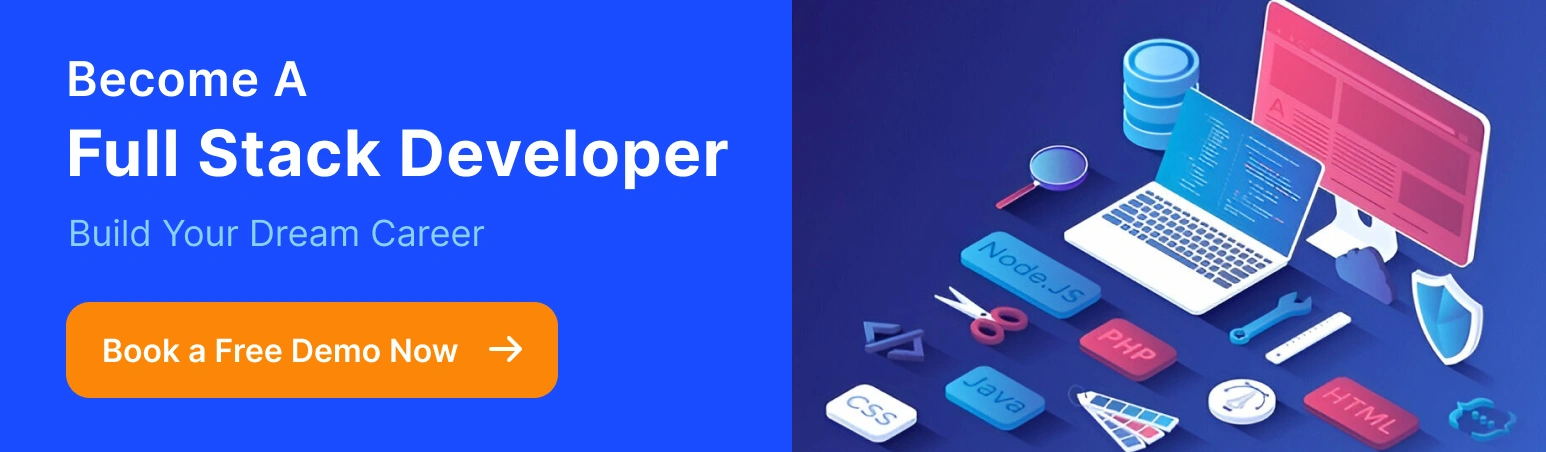
Upskill Yourself With Live Training
JavaScript Random Number Function
The Math.random() function is a simple and commonly used way to generate random number in JS. This function is a part of the Math object, which provides several constants and methods for mathematical operations.
When you call Math.random(), it returns a floating-point, pseudo-random number in the range from 0 (inclusive) to 1 (exclusive). This means you will get a number like 0.5, 0.1234, etc., but never exactly 1.
So, the numbers generated by Math.random() are pseudo-random. They are generated using an algorithm and a seed (starting point), making them deterministic. This is sufficient for most non-cryptographic purposes, but it’s important to note that they are not suitable for cryptographic use due to their predictable nature.
Generate Random Number in JavaScript Between 0 and 1
Let’s generate a random number in JS using Math.random() that prints the number between 0 and 1.
JavaScript Code:
let randomDecimal = Math.random();
console.log(randomDecimal);
Output:
0.7381904799295932
Explanation:
The Math.random() function is called, and its result is stored in the variable randomDecimal.
This function generates a pseudo-random floating-point number between 0 (inclusive) and 1 (exclusive).
The console.log statement prints the generated number to the console. In this case, the output is 0.7381904799295932. Keep in mind that each time you run this code, Math.random() will generate a different number.
Uses: You can use this program as-is for cases where a random decimal is needed, or it can be further processed to obtain random integers or random numbers within a different range, as per your requirements.
How to Generate Random Number in JavaScript Within Range?
generate random number in javascript within a range
Now, if you want to generate random number in JavaScript within a range of any specified numbers, then use the following program:
JavaScript Code:
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1) + min);
}
let randomInt = getRandomInt(20, 60);
console.log(randomInt);
Output:
45
Explanation:
We have defined the getRandomInt function to take two parameters, min and max, representing the lower and upper bounds of the desired range (inclusive).
The Math.ceil(min) ensures that the lower bound is rounded up to the nearest integer, and Math.floor(max) rounds down the upper bound.
The expression Math.floor(Math.random() * (max – min + 1) + min) generates a random integer within the given range.
The console.log statement prints the generated number to the console.
In this example, we have given the range of 20 and 60, and the random number in output is 45. You can change the range as per your requirements.
Uses: This method is useful when you need a random integer within a specific range, such as for games, random quizzes, or any application requiring a random selection from a set of options.
JavaScript Random Number Between 1 and 10
Below is an example of how to generate a random integer in JavaScript between 1 and 10 using Math.random():
JavaScript Code:
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1) + min);
}
let randomInt = getRandomInt(1, 10);
console.log(randomInt);
Output:
8
Explanation:
We are using a function named getRandomInt that generates a random integer within a specified range, inclusive of both the minimum (min) and maximum (max) bounds.
The function adjusts the min and max values to ensure they are integers and then calculates a random integer between these values using the expression Math.floor(Math.random() * (max – min + 1) + min).
The console.log statement prints the generated random integer to the console.
Uses: This method is commonly used in scenarios where a random integer within a small, defined range is needed, such as in basic games, random selections, or simple simulations.
JavaScript Random Number Between 1 and 100
Next, we are writing a program to generate a random number in JavaScript where the number will be between 1 and 100.
JavaScript Code:
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1) + min);
}
let randomInt = getRandomInt(1, 100);
console.log(randomInt);
Output:
58
Explanation:
This code snippet defines a function getRandomInt, which generates a random integer within a specified range. It takes two arguments, min and max, which are the lower and upper bounds of the range, respectively.
The Math.ceil(min) ensures the lower bound is an integer, and Math.floor(max) does the same for the upper bound. The main expression inside the function, Math.floor(Math.random() * (max – min + 1) + min), computes a random integer within the range [min, max].
The console.log statement outputs the random integer.
Generate 6 Digit Random Number in JavaScript
Below is an example of how to generate a 6-digit random number in JavaScript using Math.random(), along with the simulated output and a brief explanation:
JavaScript Code:
function getRandomSixDigit() {
return Math.floor(Math.random() * (999999 - 100000 + 1)) + 100000;
}
let randomSixDigit = getRandomSixDigit();
console.log(randomSixDigit);
Output:
111447
Explanation:
The function getRandomSixDigit generates a random integer within the range of 100000 (inclusive) and 999999 (inclusive). This is achieved by scaling the output of Math.random() to the desired range.
The expression Math.floor(Math.random() * (999999 – 100000 + 1)) + 100000 ensures that the random number generated is always a 6-digit integer.
Uses: This method is useful in scenarios where a fixed-length random number is required, such as for generating unique user IDs, verification codes, or for certain types of random sampling.
Generate 4 Digit Random Number in JavaScript
Like the above program, we can also generate a 4-digit random number in JavaScript using Math.random():
JavaScript Code:
function getRandomFourDigit() {
return Math.floor(Math.random() * (9999 - 1000 + 1)) + 1000;
}
let randomFourDigit = getRandomFourDigit();
console.log(randomFourDigit);
Output:
7693
Explanation:
The getRandomFourDigit function generates a random integer within the range of 1000 (inclusive) to 9999 (inclusive). This is accomplished by scaling the output of Math.random() to the specified range.
The expression Math.floor(Math.random() * (9999 – 1000 + 1)) + 1000 ensures that the random number generated is always a 4-digit integer.
Uses: This function is particularly useful in situations where a fixed-length random number is needed, like pin codes, simple authorization codes, or as part of a larger randomization process in applications.
Generate 10 Digit Random Number in JavaScript
Below is an example of how to generate a 10-digit random number in JavaScript:
JavaScript Code:
function getRandomTenDigit() {
return Math.floor(Math.random() * (9999999999 - 1000000000 + 1)) + 1000000000;
}
let randomTenDigit = getRandomTenDigit();
console.log(randomTenDigit);
Output:
8010521021
Explanation:
The getRandomTenDigit function is designed to generate a random integer within the range of 1000000000 (inclusive) to 9999999999 (inclusive).
The expression Math.floor(Math.random() * (9999999999 – 1000000000 + 1)) + 1000000000 accomplishes this by scaling and shifting the output of Math.random() to the desired 10-digit range.
Uses: This method is useful in scenarios requiring a long, fixed-length random number, such as generating unique identifiers, transaction reference numbers, or for specific random sampling applications in larger systems.
JS Random Number Projects for Practice
Now that you know how to get a random number in JS, you must put your skills to test by creating these web development projects:
1. Dice Roll Simulation
Project Idea: Create a simple web application that simulates the rolling of one or more dice.
Key Concepts: Generating random numbers within a specific range (1 to 6 for a standard die), DOM manipulation to display results.
Extension: Allow users to choose the number of dice and display the sum of the rolled values.
2. Random Color Generator
Project Idea: Develop an application that generates and displays a random color every time the user clicks a button.
Key Concepts: Generating random numbers for RGB values (0-255), CSS manipulation to change background colors.
Extension: Display the RGB or hexadecimal color code on the screen.
3. Random Quote Generator
Project Idea: Create a web page that displays a random inspirational quote from a predefined list every time a user clicks a button.
Key Concepts: Array manipulation, accessing array elements randomly.
Extension: Fetch quotes from an external API for a larger collection of quotes.
4. Password Generator
Project Idea: Build a tool that generates a secure random password based on user-selected criteria (length, inclusion of numbers/symbols).
Key Concepts: String manipulation, generating random alphanumeric characters.
Extension: Add options to include/exclude specific character sets (like special characters, uppercase, etc.).
5. Flashcard Quiz Game
Project Idea: Create a simple flashcard-style quiz game where questions are presented randomly.
Key Concepts: Array shuffling, basic event handling for user interactions.
Extension: Track and display the user’s score or add time limits for answering.
6. Random Name Picker
Project Idea: Develop an application that randomly selects a name from a list, useful for decision making, games, or classroom use.
Key Concepts: Handling user input, random selection from an array.
Extension: Allow users to add, remove, and save names to the list.
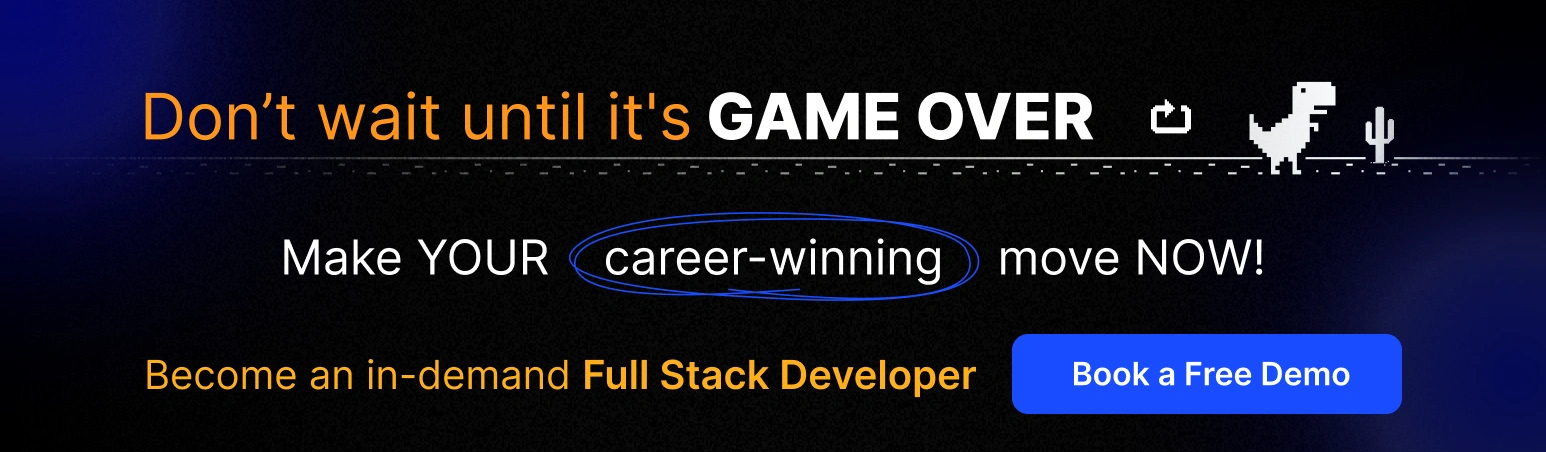
Got the Programs? Challenge Yourself With This Quiz!
1. What does the JavaScript function Math.random() return?
a) An integer between 0 and 1.
b) A floating-point number between 0 (inclusive) and 1 (exclusive).
c) A floating-point number between 0 and 10.
d) A random color code.
2. Which of the following is true about the numbers generated by Math.random()?
a) They are cryptographically secure.
b) They are pseudo-random.
c) They can be seeded.
d) They are always integers.
3. How can you generate a random integer between 1 and 10 using Math.random()?
a) Math.random() * 10 + 1
b) Math.floor(Math.random() * 10) + 1
c) Math.ceil(Math.random() * 10)
d) Math.round(Math.random() * 10)
4. What is the purpose of using Math.floor() in random number generation?
a) To convert a decimal number to its nearest lower integer.
b) To create a floor plan using random numbers.
c) To generate a floating-point number.
d) To round a number to its nearest higher integer.
5. Can you set a seed for the Math.random() function in JavaScript?
a) Yes, using the Math.seed() function.
b) Yes, by passing a seed value as an argument to Math.random().
c) No, JavaScript does not provide a way to seed Math.random().
d) No, because Math.random() always generates a fixed sequence of numbers.
6. Which of the following scenarios is NOT suitable for using Math.random()?
a) Generating a random color for a website background.
b) Creating a secure cryptographic key.
c) Shuffling a list of quiz questions.
d) Picking a random number for a dice roll in a game.
7. What would be a good use case for a custom random number generator function in JavaScript?
a) Generating a random number between 0 and 1.
b) Creating a random alphanumeric string.
c) Calculating mathematical equations.
d) None of the above.
8. Which method is commonly used to scale Math.random() output to a specific range?
a) Multiplication and addition.
b) Subtraction and division.
c) Logarithmic scaling.
d) Exponential transformation.
Answer Key:
- b
- b
- b
- a
- c
- b
- b
- a
Try interactive JavaScript Quiz!
More Web Development Quizzes:
FAQs About Generating Random Numbers in JS
Math.random() generates a floating-point, pseudo-random number in the range from 0 (inclusive) to 1 (exclusive). This means you’ll get numbers like 0.5, 0.1234, but never 1.
No, Math.random() is not suitable for cryptographic purposes because it is not cryptographically secure. For cryptographic applications, it’s better to use more secure methods like the Web Crypto API.
You can generate a random boolean by using Math.random() and checking if it is greater or less than 0.5, like this: Math.random() < 0.5.
JavaScript does not provide a built-in method to set a seed for its random number generator. This is by design, as Math.random() is meant to provide quick and basic pseudo-random numbers without the complexity of seed management.
To create a random string, you can first define a string of all possible characters, and then repeatedly use Math.random() to pick a character from this string until you reach your desired length.
Yes, there are several third-party libraries available for advanced random number generation in JavaScript, such as Chance.js, Math.js, and others that offer additional functionality like seeded random number generation and more.
Wrapping Up:
Understanding how to generate random numbers in JavaScript is a stepping stone in your journey as a web developer. While this post has provided you with the basics and practical applications, there’s a wide range of web development concepts waiting to be explored.
Go for a live online JavaScript course or a full stack developer course for a more comprehensive learning experience. These courses not only deepen your understanding but also equip you with the skills needed to build a robust career in technology.
Upskilling through structured learning undoubtedly opens new doors and presents exciting career opportunities in web development.
Happy Coding!
Learn more JS programs