Finding the square root in JavaScript is an interesting concept that brings together mathematics and web development. We all have been learning to find the square root of a number since our school days, and this mathematical concept doesn’t leave you alone, even if you want to learn web development.
But, here is a catch. You no longer need to calculate it manually. All you need to do is to write a JavaScript program to find the square root. This program will solve all kinds of square roots within seconds.
For those who don’t remember: The square root of a number is a value that, when multiplied by itself, gives the original number. For example, the square root of 9 is 3, because 3 × 3 = 9.
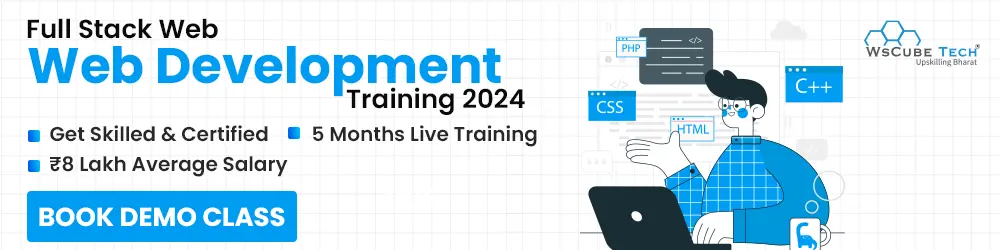
So, let’s get started and master the JavaScript square root program along with core concepts.
Before that… if you are a beginner or student looking to build a high-paying career in development, then you must book the free live class of the online Full Stack Developer Course by WsCube Tech.
Why Use Square Root in JavaScript?
The role of using a JavaScript program to calculate square roots in web development is multifaceted and important. It is especially useful in enhancing the functionality and interactivity of web applications.
Let’s know how JavaScript square root program helps in web development:
- Data Processing and Analysis: Many web applications deal with data that requires mathematical processing. Whether it’s for financial calculations, scientific data analysis, or even in gaming applications, the ability to compute square roots is a basic yet essential part of these processes.
- Enhancing User Experience: Interactive web applications need to perform quick calculations on the client side to provide instant feedback or results to the user. JavaScript, being the backbone of client-side scripting, can efficiently execute square root calculations without the need for server-side processing, leading to a smoother user experience.
- Educational Applications: For websites focused on education, particularly in subjects like mathematics, physics, or engineering, a square root calculation function is fundamental. Implementing this in JavaScript allows for interactive learning tools, where students can input values and instantly see results.
- Financial Applications: In financial web apps, such as loan calculators or investment projection tools, JS square root functions can be used in various financial formulas and models, including statistical calculations and risk assessments.
- Graphics and Visualizations: JavaScript is widely used in creating graphics and animations on the web. Calculating square roots can be crucial in certain types of visual representations, such as plotting points on a graph or creating shapes and animations with geometric precision.
Upskill Yourself With Live Training
JavaScript Square Root Function
JavaScript provides us with a built-in function to calculate the square root. The name of JavaScript square root function is Math.sqrt().
Math.sqrt() function in JavaScript, part of the Math object, serves as a collection of mathematical constants and functions. It is specifically used to calculate the square root of a given number in JS.
Syntax:
The syntax of JavaScript square root function is quite simple:
Math.sqrt(number);
Here, number is the value whose square root you want to find. This value must be a positive number or zero, as square roots of negative numbers aren’t real numbers in the basic numeric system used in JavaScript.
If the input is positive or zero, the function returns the square root of the given number. If the input is negative, Math.sqrt() returns NaN, which stands for “Not-a-Number”, indicating an undefined or unrepresentable value in JavaScript.
- The Math.sqrt() function is highly reliable and provides a precise square root value up to the limits of the JavaScript Number precision.
- Being a built-in function, it saves programmers from the complexity of implementing square root logic manually. It’s straightforward and can be used without importing any external libraries.
JavaScript Program to Find Square Root
Now, let’s create a simple JavaScript program to find the square root of a number using the built-in Math.sqrt() function.
JavaScript Code:
// Prompt the user to enter a number
const number = prompt('Enter the number: ');
// Check if the input is a number
if(!isNaN(number)) {
// Calculate the square root
const result = Math.sqrt(number);
console.log(`The square root of ${number} is ${result}`);
} else {
console.log('Please enter a valid number');
}
Output:
Enter the number: 81
The square root of 81 is 9
Explanation:
- const number = prompt(‘Enter the number: ‘);
The program begins by displaying a prompt dialog box to the user, asking them to enter a number. This is done using the prompt() function, which is a built-in browser function that captures user input.
- if(!isNaN(number)) { … } else { … }
Before proceeding with the square root calculation, the program checks if the entered value is actually a number. This is important because prompt() returns a string, and the user might enter non-numeric values. The isNaN() function is used to check if the input is ‘Not-a-Number’. If isNaN(number) returns true, it means the input is not a number; the ! operator negates this, so the if block executes when the input is a number.
- const result = Math.sqrt(number);
Inside the if block, assuming the input is a valid number, the program calculates the square root of the entered number using the Math.sqrt() function.
- console.log(The square root of ${number} is ${result});
After calculating the square root in JavaScript, the result is displayed in the console. The program uses template literals (enclosed in backticks `) to create a string that includes the entered number and its square root.
Template literals allow embedded expressions, which is used here to insert the values of numberandresult` into the string.
- console.log(‘Please enter a valid number’);
If the input is not a valid number, the else block executes, and the program logs a message to the console asking the user to enter a valid number. This helps in providing feedback to the user in case of incorrect input.
More JavaScript Programs for You to Practice:
How to Find Square Root in JavaScript Without Using Function?
In case you want to find the square root of a number without using the in-built function, then you need to manually implement a square root calculation. For instance, you can use the iterative method like the Babylonian method (also called Heron’s method).
This method approximates the square root of a number by using a guess-and-check approach.
JavaScript Code:
// JavaScript Program to Calculate Square Root Without Function
function findSquareRoot(number) {
let guess = number;
const accuracy = 0.000001; // This determines the accuracy of the result
while ((guess - number / guess) > accuracy) {
guess = (guess + number / guess) / 2;
}
return guess;
}
// Example usage
let number = 16;
let squareRoot = findSquareRoot(number);
console.log("The square root of " + number + " is " + squareRoot);
Output:
The square root of 16 is 4.000000000000051
Explanation:
- We define findSquareRoot(number) which takes a number as its argument.
- We start with an initial guess for the square root. A good starting point is the number itself (let guess = number).
- We define an accuracy variable to decide how close our guess needs to be to the actual square root. The smaller the value, the more accurate our result (but it might take more iterations).
- The main logic is in the while loop. We repeatedly adjust our guess based on the formula guess = (guess + number / guess) / 2. This formula is derived from the Babylonian method, which is an efficient way to approximate square roots.
- The loop continues until the difference between our guess squared and the original number is less than our defined accuracy.
- Once the loop is done, our guess is close enough to the actual square root, and we return this value.
- We call our findSquareRoot function with an example number (in this case, 16) and output the result using console.log.
Also Learn: Python Program to Find Square Root
Benefits of Using Math.sqrt() to Find Square Root in JavaScript
The Math.sqrt() function in JavaScript offers several advantages, making it a popular choice for calculating square roots in web development:
- Simplicity and Ease of Use: One of the top advantages of Math.sqrt() is its simplicity. The syntax is straightforward, making it accessible even to beginners. You only need to pass the number for which you want the square root, and the function handles the rest.
- Reliability: Being a built-in JavaScript function, Math.sqrt() is well-tested and reliable. It provides accurate results within the limits of JavaScript’s numerical precision.
- Efficiency: As a native JavaScript function, Math.sqrt() is optimized for performance. This efficiency is beneficial in scenarios where you need to perform a large number of square root calculations quickly, such as in real-time applications or data processing tasks.
- No Need for External Libraries: Since Math.sqrt() is built into JavaScript, there’s no need to rely on external libraries for square root calculations. This reduces the complexity of your codebase and the overhead associated with importing and managing additional dependencies.
Challenge Your Web Development Skills With Free Quizzes:
- JavaScript Quiz
- HTML Quiz
- CSS Quiz
- ReactJS Quiz
- HTML5 Quiz
- CSS3 Quiz
- PHP Quiz
- MySQL Quiz
- jQuery Quiz
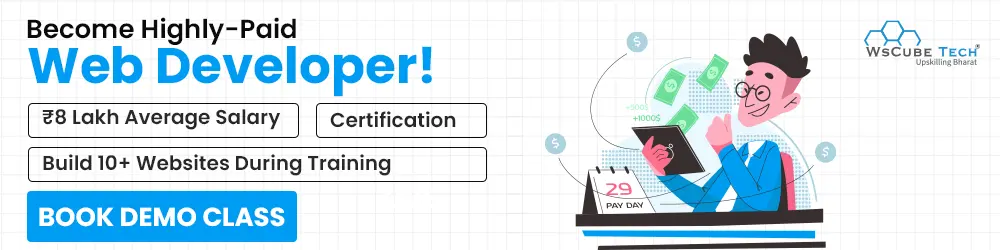
FAQs About JavaScript Square Root
Math.sqrt() is a built-in JavaScript function that is used to calculate the square root of a given number. It is a part of the Math object in JavaScript, which provides a collection of mathematical constants and functions.
No, Math.sqrt() returns NaN (Not-a-Number) when it is given a negative number as input. This is because the square root of a negative number is not a real number in the basic numerical system JavaScript uses.
Math.sqrt() is quite accurate for most practical purposes. It calculates the square root within the limits of JavaScript’s floating-point number precision. However, for extremely large or small numbers, there may be some limitations due to how JavaScript handles number precision.
Yes, you can manually implement a square root calculation using methods like the Babylonian method (also known as Heron’s method). This involves an iterative approach to approximate the square root.
Manually calculating a square root can be educational, helping you understand the underlying algorithm and improving your programming skills. It’s also useful in environments where you might need a custom implementation for specific accuracy requirements or when dealing with complex numbers.
By default, JavaScript does not support calculations involving complex numbers (numbers with a real and an imaginary part). To handle complex numbers, you would need to use an external library or implement your own complex number arithmetic.
Math.sqrt() is a highly optimized and efficient function for calculating square roots in JavaScript. It is unlikely to be a performance bottleneck in most applications. However, for applications that require a very high volume of calculations at a rapid pace, it’s always good to benchmark and test performance.
Free Courses for You
Wrapping Up:
The program to find square root of a number in JavaScript is more than just a number-crunching exercise. It is a valuable skill. We’ve looked at the simplicity and reliability of the Math.sqrt() function, and also talked about manually implementing the square root algorithm for deeper understanding.
Mastering these concepts is just the beginning of your journey in web development. If you’re eager to expand your skills further, it is highly recommended to join the live online JavaScript course by WsCube Tech.
More than that, WsCube Tech’s full stack development course is a fantastic opportunity to gain in-depth skills and hands-on experience in both front-end and back-end development, equipping you with the skills needed to become a proficient full-stack developer.
Remember, the field of web development is constantly evolving, and continuous learning is key to staying ahead.
Happy coding!