JavaScript is famous as the language of the web because it plays a crucial role in web design and development. If you are a beginner wondering how to print Hello World in JavaScript, then we have covered it here in simple terms.
This simple yet iconic Hello World program in JavaScript has been the initiation rite for countless developers, and it’s your passport to understanding the fundamentals of JS.
Whether you’re a complete beginner or just looking for a refresher, this blog post, brought to you by WsCube Tech, will equip you with the essential knowledge you need to start your web development journey.
Introduction to JavaScript
JavaScript, often known as JS, is a versatile and powerful programming language that plays a crucial role in web development. It was specifically designed to enhance the interactivity of websites, making them dynamic and responsive to user actions.
Unlike HTML and CSS, which focus on the structure and presentation of web content, JavaScript is a full-fledged programming language that enables developers to add functionality and behavior to web pages.
This means you can use JavaScript to create interactive forms, validate user input, manipulate the Document Object Model (DOM) to update page content on the go, and respond to user events like clicks and keystrokes.
JavaScript is primarily a client-side scripting language that runs directly within a user’s web browser. It allows web developers to build feature-rich web applications without relying solely on server-side processing.
This capability has made JavaScript an integral part of modern web development, powering everything from simple interactive widgets to complex web applications like social media platforms and online games.
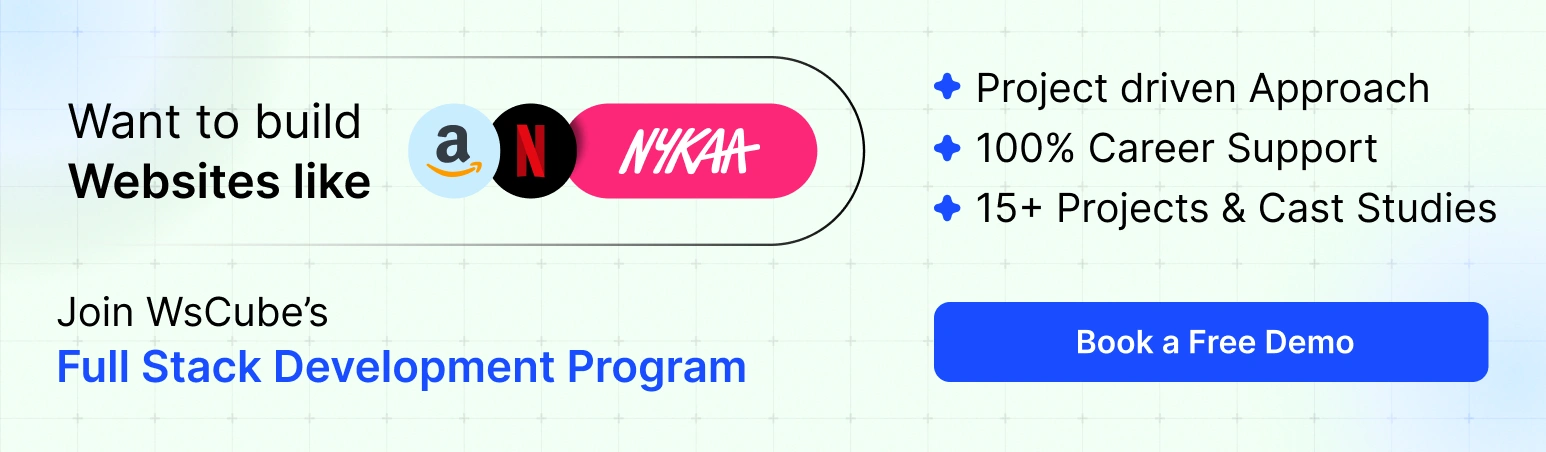
Hello World Program in JavaScript Using console.log()
The following is a program to Hello World in JavaScript using console.log(), along with the code, output, and explanation:
JavaScript Code:
// Printing hello world in JS
console.log("Hello World");
Output:
Hello World
Explanation:
- // Printing hello world in JS: This line is a comment in the code. In JavaScript, comments are denoted by two forward slashes (//) for single-line comments. Comments are ignored by the JavaScript interpreter and are used to provide explanations or documentation within the code.
- console.log(Hello World);: This line is an attempt to use the console.log() function to print “Hello World” to the console.
Print Hello World in JavaScript Using alert()
The following is a JavaScript program to print Hello World using the alert() function, along with the code, output, and explanation:
JavaScript Code:
// print hello world message
alert("Hello World");
Output:
Hello World
Explanation:
In this JS program, we use the alert() function to display the Hello World message.
- alert(“Hello World”);: This line is the core of the program. It calls the alert() function and passes the string “Hello World” as an argument.
The alert() function is a built-in JavaScript function that creates a pop-up dialog box in the browser with the specified message.
Inside the parentheses (), we provide the message we want to display, which is “Hello World” in this case.
Important Things to Know About This JS Program:
- The alert() function is commonly used for simple notifications or alerts in JavaScript.
- Using alert() to display messages can be intrusive and might disrupt the user experience on a webpage. For this reason, it’s often used sparingly and mainly for debugging or displaying critical information.
- Unlike console.log(), which is used for debugging and doesn’t interrupt the user’s interaction with the webpage, alert() requires user interaction to dismiss the pop-up.
This method to print Hello World in JavaScript is generally used for specific scenarios where user attention is required.
Print Hello World in JS Using document.write()
The following is a program to print Hello World in JavaScript using the document.write() method, along with the code, output, and explanation:
JavaScript Code:
// hello world program in js
document.write("Hello World");
Output:
Hello World
Explanation:
Here, we use the document.write() method to write the “Hello World” message directly to the web page.
- document.write(“Hello World”);: This line is the core of the program. It calls the document.write() method and passes the string “Hello World” as an argument.
The document.write() method is used to write content to the document (web page).
Inside the parentheses (), we provide the message we want to write, which is “Hello World” in this case.
Things to Know:
- The document.write() method is a way to dynamically generate content within an HTML document using JavaScript.
- Using document.write() can have side effects. When called after the HTML document has fully loaded, it can overwrite the entire content of the page. This behavior should be used cautiously.
- If you use document.write() after the document has been fully loaded, it will replace the entire content of the webpage with the specified message. In practice, it’s often used during the initial page load or in specific scenarios where you want to add dynamic content.
JavaScript Hello World Program: Video
Common Mistakes You Might Face and Solutions
As a beginner, you might face some common mistakes while learning and writing the JavaScript Hello World program. These mistakes are part of the learning process, and understanding how to identify and fix them is crucial for progress.
1. Syntax Errors
- Mistake: Missing semicolons, incorrect capitalization, or typos in variable or function names can lead to syntax errors.
- How to Fix: Carefully review your code, pay attention to proper syntax, and use code editors with syntax highlighting to catch errors early. Debugging tools in browsers can also help identify syntax issues.
2. Undefined Variables
- Mistake: Referencing a variable before it’s declared or trying to use a variable that doesn’t exist.
- How to Fix: Ensure variables are declared before use, and double-check variable names for typos. Use let, const, or var to declare variables.
3. Misusing Quotes
- Mistake: Not using quotes around strings or mixing single and double quotes incorrectly.
- How to Fix: Always enclose strings in single (‘) or double (“) quotes consistently. If you need to include quotes within a string, use the opposite type for the string’s delimiters.
4. Improper Indentation
- Mistake: Inconsistent or incorrect indentation makes code hard to read and understand.
- How to Fix: Use consistent indentation (e.g., 2 or 4 spaces) for better readability. Most code editors can help auto-indent your code.
5. Confusing Assignment and Comparison Operators
- Mistake: Using = instead of == or === for comparison, leading to unexpected behavior.
- How to Fix: Use = for assignment and == or === for comparisons. Be aware of the difference between equality (==) and strict equality (===).
6. Not Using var, let, or const
- Mistake: Failing to declare variables using var, let, or const.
- How to Fix: Always declare variables with var, let, or const to avoid unexpected global scope issues.
7. Missing Parentheses or Curly Braces
- Mistake: Omitting parentheses for function calls or curly braces for blocks can result in syntax errors or unintended behavior.
- How to Fix: Ensure proper use of parentheses for function calls and curly braces for code blocks.
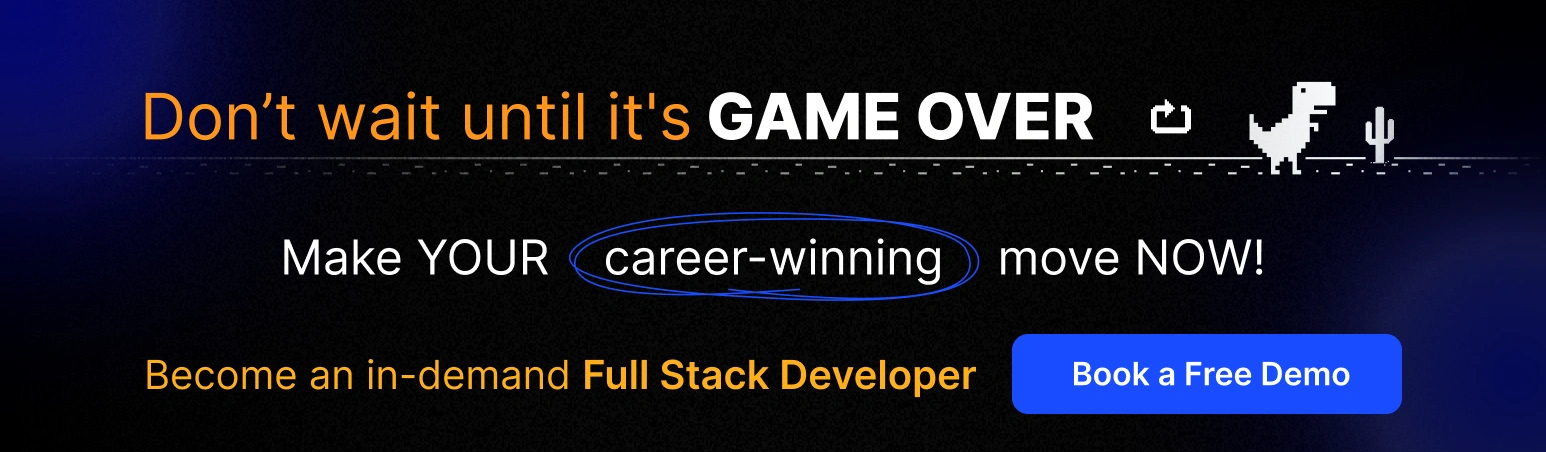
FAQs About JavaScript Hello World Program
A Hello World program in JavaScript is a simple introductory program that displays the message “Hello World”.
A Hello World program is the first step in learning a new programming language. It helps beginners get familiar with the basic syntax and structure of the language.
console.log() is used for debugging and logging messages to the browser’s console, while alert() is used to display a pop-up dialog to the user. console.log() is generally used for development, while alert() is for user interactions or notifications.
Yes, you can customize the message to anything you like. For example, you can use console.log(“Welcome to WsCube Tech!”); to display a custom message.
Wrapping Up:
This beginner’s guide to writing a Hello World program in JavaScript marks the exciting start of your web development career. As you explore JavaScript’s fundamentals, remember to take your skills further with the Full Stack Developer Course by WsCube Tech. Visit the linked course page for details and book your live demo class.
Read more blogs