JavaScript is one of the core technologies of web development. It is a versatile and powerful language used for everything from simple web page interactions to complex web applications. Learning it is key to becoming a proficient web developer.
In this tutorial, we will learn how to add two numbers in JavaScript, along with code examples, output, and simple explanations.
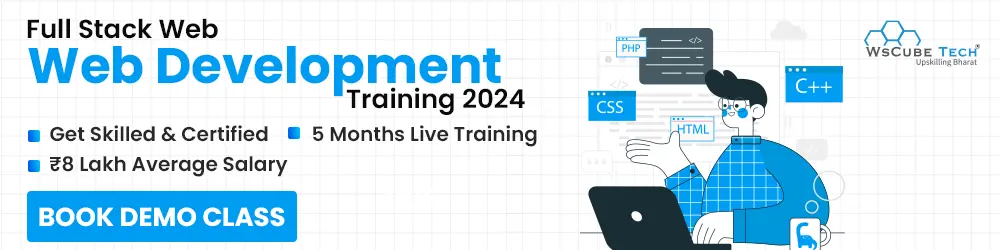
Add Two Numbers in JavaScript Using + Operator
Below is a simple JavaScript program to add two numbers using the + operator, along with explanation:
JavaScript Code:
// Declare two variables and assign numbers to them
var number1 = 5;
var number2 = 3;
// Add the two numbers using the + operator
var sum = number1 + number2;
// Print the result
console.log("The sum of the two numbers is: " + sum);
Output:
The sum of the two numbers is: 8
Explanation
- var number1 = 5; and var number2 = 3;: Here, we declare two variables named number1 and number2. Variables are like containers that store data values. In this case, we store the numbers 5 and 3 in these containers, respectively.
- var sum = number1 + number2;: This line creates another variable called sum. The + operator is used to add the values of number1 and number2. The result of this addition (5 + 3) is stored in the sum variable.
- console.log(“The sum of the two numbers is: ” + sum);: This line is used to print the result. console.log() is a function that prints the specified message to the console. Here, it concatenates (joins together) the string “The sum of the two numbers is: ” with the value of the sum variable (8) and prints the complete message.
Upskill Yourself With Live Training
Recommended Courses for You | Book Live Class for FREE! |
Full Stack Web Development Course | Book Now |
WordPress Course | Book Now |
Front-End Development Course | Book Now |
Add Two Numbers in JavaScript Using Function
Now, we will write a JavaScript program to add two numbers using function:
JavaScript Code:
// Function to add two numbers
function addNumbers(num1, num2) {
return num1 + num2;
}
// Declare two numbers
var number1 = 5;
var number2 = 10;
// Call the function and store the result
var sum = addNumbers(number1, number2);
// Display the result
console.log("The sum of " + number1 + " and " + number2 + " is: " + sum);
Output:
The sum of 5 and 10 is: 15
Explanation:
- function addNumbers(num1, num2) { … }: This is a function named addNumbers. Functions are reusable blocks of code that perform a specific task. Here, the task is to add two numbers. The num1 and num2 inside the parentheses are parameters, which are variables that act as placeholders for the values you want to add.
- return num1 + num2;: Inside the function, the return statement is used to send back, or return, the result of num1 + num2. This allows the function to produce an output.
- var number1 = 5; var number2 = 10;: These lines declare two variables number1 and number2 and assign them the values 5 and 10, respectively.
- var sum = addNumbers(number1, number2);: Here, the addNumbers function is called with number1 and number2 as arguments (actual values passed to the function). The function calculates the sum and returns it. This returned value is then stored in another variable called sum.
- console.log(“The sum of ” + number1 + ” and ” + number2 + ” is: ” + sum);: This line prints out the result. It uses console.log() to display the string with the values of number1, number2, and sum concatenated together.
Addition of Two Numbers in JavaScript Using Addition Assignment (+=) Operator
The third method is to add two numbers in JS using the addition assignment operator (+=). The += operator in JavaScript is useful for adding a value to a variable and then updating that variable with the new value.
JavaScript Code:
// Declare a variable for the sum and initialize it to 0
var sum = 0;
// Declare two numbers to be added
var number1 = 5;
var number2 = 10;
// Use the += operator to add number1 to sum
sum += number1; // sum is now 5
// Use the += operator to add number2 to sum
sum += number2; // sum is now 15
// Display the result
console.log("The sum of " + number1 + " and " + number2 + " is: " + sum);
Output:
The sum of 5 and 10 is: 15
Explanation:
- var sum = 0;: This line declares a variable named sum and initializes it with the value 0. This variable will hold the total sum of the numbers.
- var number1 = 5; var number2 = 10;: These lines declare two variables, number1 and number2, and assign them the values 5 and 10, respectively. These are the numbers we want to add.
- sum += number1;: The += operator is a shorthand for adding a value to a variable. This line takes the current value of sum (which is 0), adds number1 (which is 5) to it, and then updates sum to this new value (so sum becomes 5).
- sum += number2;: Similarly, this line adds number2 (which is 10) to the current value of sum (which is 5), resulting in sum becoming 15.
- console.log(“The sum of ” + number1 + ” and ” + number2 + ” is: ” + sum);: This line uses the console.log() function to print out the final sum.
JavaScript Program to Add Two Numbers Using Arrow Function
Below is a program in JavaScript to add two numbers using an arrow function. The arrow functions provide a more concise syntax for writing functions and are particularly useful for simple operations. They are an important part of modern JavaScript and a useful concept for beginners to learn.
JavaScript Code:
// Arrow function to add two numbers
const addNumbers = (num1, num2) => num1 + num2;
// Declare two numbers
const number1 = 5;
const number2 = 10;
// Call the arrow function and store the result
const sum = addNumbers(number1, number2);
// Display the result
console.log("The sum of " + number1 + " and " + number2 + " is: " + sum);
Output:
The sum of 5 and 10 is: 15
Explanation:
- const addNumbers = (num1, num2) => num1 + num2;: This is an arrow function, a concise way to write functions in JavaScript. It starts with the const keyword to declare a constant named addNumbers. The parameters num1 and num2 are placed inside parentheses (), followed by the arrow =>, and then the expression that defines what the function does. This expression simply adds num1 and num2.
- const number1 = 5; const number2 = 10;: These lines declare two constants number1 and number2, and assign them the values 5 and 10, respectively. const is used to declare variables whose values are not intended to change.
- const sum = addNumbers(number1, number2);: This line calls the addNumbers arrow function with number1 and number2 as arguments. The function calculates the sum of these numbers and returns it. The returned value is stored in a constant called sum.
- console.log();: This line prints out the result to the console. It concatenates the string with the values of number1, number2, and sum.
Add Two Numbers in JavaScript (User Input)
Creating a JavaScript program to add two numbers based on user input involves getting input through a method like prompt(), which is used in a browser environment. This method displays a dialog box that prompts the user for input.
JavaScript Code:
// Function to add two numbers
function addNumbers(num1, num2) {
return num1 + num2;
}
// Get user input
var number1 = parseFloat(prompt("Enter the first number:"));
var number2 = parseFloat(prompt("Enter the second number:"));
// Check if the inputs are numbers
if (isNaN(number1) || isNaN(number2)) {
console.log('Please enter valid numbers');
} else {
// Call the function and display the result
var sum = addNumbers(number1, number2);
console.log("The sum of " + number1 + " and " + number2 + " is: " + sum);
}
Output:
Enter the first number:30
Enter the second number:463
The sum of 30 and 463 is: 493
Explanation:
- prompt(“Enter the first number:”): This displays a dialog box asking the user to enter the first number. The entered value is then converted from a string to a floating-point number using parseFloat().
- Similarly, the program gets the second number using another prompt().
- It checks if the entered values are valid numbers using isNaN(). If either value is not a number, it logs an error message.
- If both inputs are valid, it calculates the sum using the addNumbers function and logs the result to the console.
Interview Questions for You to Prepare for Jobs
JavaScript Program to Add Two Numbers Using textbox
The program to add two numbers in JavaScript using textbox includes HTML for the input fields and JavaScript to handle the input and display the output.
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<title>Add Two Numbers Using Textboxes</title>
</head>
<body>
<!-- Textboxes for user input -->
<input type="text" id="number1" placeholder="Enter first number">
<input type="text" id="number2" placeholder="Enter second number">
<!-- Button to trigger the calculation -->
<button onclick="addNumbers()">Calculate Sum</button>
<!-- Element to display the result -->
<p id="result"></p>
<script>
// Function to add numbers
function addNumbers() {
// Get the values from the textboxes and convert them to numbers
var num1 = parseFloat(document.getElementById('number1').value);
var num2 = parseFloat(document.getElementById('number2').value);
// Check if the inputs are numbers
if (isNaN(num1) || isNaN(num2)) {
document.getElementById('result').innerHTML = 'Please enter valid numbers';
return;
}
// Add the numbers
var sum = num1 + num2;
// Display the result
document.getElementById('result').innerHTML = 'The sum is: ' + sum;
}
</script>
</body>
</html>
Output:
[Textbox] "Enter first number"
[Textbox] "Enter second number"
[Button] "Calculate Sum"
After entering 7 in the first box and 3 in the second box, and clicking the “Calculate Sum” button:
[Textbox] "7"
[Textbox] "3"
[Button] "Calculate Sum"
"The sum is: 10"
If non-numeric values are entered:
[Textbox] "abc"
[Textbox] "xyz"
[Button] "Calculate Sum"
"Please enter valid numbers"
Explanation:
<input type=”text” id=”number1″> and <input type=”text” id=”number2″> create two textboxes for users to enter numbers. The id attribute is used to uniquely identify each textbox.
<button onclick=”addNumbers()”>Calculate Sum</button> creates a button. When clicked, it calls the addNumbers() function defined in the JavaScript.
<p id=”result”></p> is a paragraph tag where the sum will be displayed.
function addNumbers() { … } is a JavaScript function that gets executed when the button is clicked.
parseFloat(document.getElementById(‘number1’).value) and parseFloat(document.getElementById(‘number2’).value) retrieve the values entered in the textboxes, converting them to floating-point numbers.
The isNaN function checks if the inputs are valid numbers. If not, an error message is displayed.
var sum = num1 + num2; calculates the sum of the two numbers.
document.getElementById(‘result’).innerHTML = ‘The sum is: ‘ + sum; updates the paragraph content to show the result.
How to Add Two Numbers in JavaScript Dynamically?
Adding two numbers dynamically in JavaScript includes interacting with HTML elements to get user input, performing the addition in JavaScript, and then displaying the result back on the webpage. This dynamic interaction makes the webpage responsive to user actions.
HTML Structure:
First, you’ll need an HTML structure with input fields for the numbers, a button to trigger the calculation, and an element to display the result.
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Addition in JavaScript</title>
</head>
<body>
<!-- Input fields for the numbers -->
<input type="number" id="number1" placeholder="Enter first number">
<input type="number" id="number2" placeholder="Enter second number">
<!-- Button to trigger the addition -->
<button onclick="addNumbers()">Add Numbers</button>
<!-- Element to display the result -->
<p id="result"></p>
<script>
// JavaScript code will go here
</script>
</body>
</html>
JavaScript Code:
Inside the <script> tag, you’ll write the JavaScript function that reads the input values, adds them, and displays the result.
function addNumbers() {
// Retrieve the values from the input fields
var num1 = parseFloat(document.getElementById('number1').value) || 0;
var num2 = parseFloat(document.getElementById('number2').value) || 0;
// Add the numbers
var sum = num1 + num2;
// Display the result
document.getElementById('result').innerHTML = 'The sum is: ' + sum;
}
Explanation:
HTML Inputs: The <input type=”number”> fields allow users to enter numbers. The id attributes (number1 and number2) are used to reference these elements in JavaScript.
Button: The <button> element has an onclick attribute that calls the addNumbers() JavaScript function when clicked.
Result Display: The <p id=”result”> paragraph will display the sum.
JavaScript Function: addNumbers() is the function that gets called when clicking the button.
It uses document.getElementById().value to retrieve the values from the input fields.
parseFloat() converts the input values to floating-point numbers. The || 0 ensures that if the input field is empty, it treats it as zero.
It calculates the sum and then updates the inner HTML of the result paragraph to display the sum.
Also read: Hello World Program in JavaScript (3 Ways With Code)
Concepts Used in Above Programs to Add Two Number in JS
The JavaScript programs above show several fundamental concepts that are crucial for beginners to understand:
1. Variables
Variables are used to store data values. In JavaScript, var, let, and const are used to declare variables. var is function-scoped, while let and const are block-scoped. const is used for variables whose values are not intended to be reassigned.
2. Data Types
JavaScript has different data types like Number, String, Boolean, Object, etc. In the programs above, we primarily dealt with Numbers (for arithmetic) and Strings (for displaying text).
3. Operators
Arithmetic Operators: Such as + for addition.
Assignment Operators: Such as = for assigning values to variables, and += for adding a value to a variable and then updating it.
4. Functions
Functions are blocks of code designed to perform a particular task. They are executed when something invokes (calls) them. The function keyword is used to define a function in JavaScript.
5. Arrow Functions
Introduced in ES6, arrow functions (=>) offer a more concise syntax for writing functions. They are especially useful for simple functions and when working with higher-order functions.
6. Event Handling
JavaScript can execute code in response to events like mouse clicks, form submissions, etc. In the examples, the onclick attribute in the button element is used to call a JavaScript function.
7. Type Conversion
JavaScript is a loosely typed language, which means variables can be converted from one type to another. Functions like parseFloat convert strings to floating-point numbers.
8. Input Validation
Checking if the input is valid before processing it. Using isNaN() (not shown in the examples but important) checks if a value is not a number.
9. Console Logging
console.log() is used for debugging purposes. It outputs a message to the web console.
10. String Concatenation
This is the process of joining two or more strings together. In JavaScript, it can be done using the + operator.
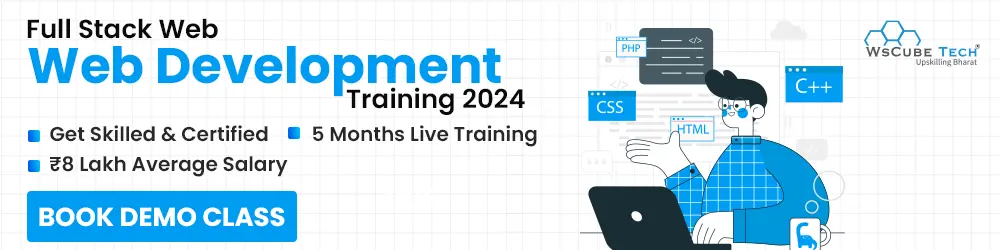
FAQs About Addition in JS
Learning to add numbers is a fundamental programming skill. It helps you understand basic JavaScript operations, variable handling, and user input processing, which are crucial for more complex programming tasks.
Yes, you can use various types of input fields, such as text or number. However, ensure you properly convert the input values to numbers using methods like parseInt() or parseFloat() before performing arithmetic operations.
The program should ideally handle such cases. In the provided examples, the program checks if the inputs are valid numbers and displays an error message if they are not.
Absolutely! You can modify the function to accept more parameters or use an array to pass multiple numbers, iterating over the array to calculate the sum.
Yes, you can create a running total by storing the sum in a variable outside the function that adds the numbers. Each time a new number is added, update this variable and display the updated total.
You can enhance interactivity by adding features like real-time result display as the user types the numbers, using events like onkeyup in JavaScript.
JavaScript uses floating-point arithmetic, which can sometimes lead to precision issues with very large or small numbers. It’s good to be aware of these limitations when performing arithmetic operations.
Free Courses for You
Course Name | Course Name |
Google Tag Manager Course | Affiliate Marketing Course |
Semrush Course | Video Editing Course |
Blogging Course | Email Marketing Course |
Shopify Course | Photoshop Course |
Wrapping Up:
Hope this tutorial helped you learn how to add two numbers in JavaScript with different approaches and according to use cases. If you aspire to build your career as a web developer, you must book a free demo class of the best full stack developer course by WsCube Tech. It comes with expert-led live training, regular classes, dedicated doubt sessions, certifications, career guidance, and more.
Read more blogs