In our series of JavaScript programs, today we are going to learn about the factorial program in JS. It is a classic problem in both coding and mathematics.
Before understanding how to find factorial of a number in JavaScript, let’s first clarify what exactly is the meaning of factorial.
In maths, the factorial of a number (let’s say N) is the product of all positive integers less than or equal to the given number (N).
For example, the factorial of 5 (denoted as 5!) is 5 × 4 × 3 × 2 × 1, which equals 120. This concept isn’t just a mathematical curiosity; it’s fundamental in various areas of computer science, from algorithms to data analysis.
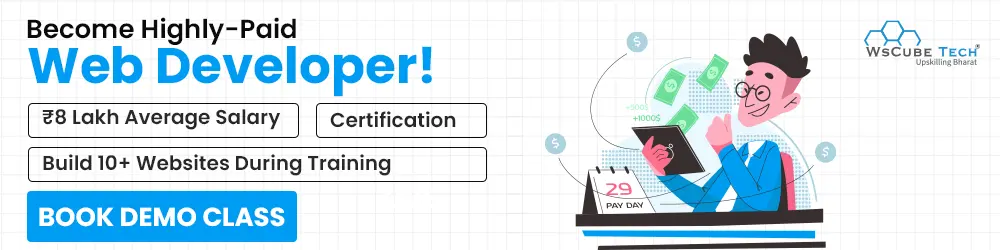
Learning the factorial program in JavaScript is more than just learning a specific task. It’s a window into essential programming concepts such as loops, recursion, and higher-order functions. These are the building blocks that every aspiring web developer needs to master. And as JavaScript continues to be a cornerstone in web development, grasping these concepts is more relevant than ever.
And, if you are passionate about web development, book the live demo class of the online Full Stack Developer course by WsCube Tech. This training program is designed to equip you with a well-rounded skill set, from front-end technologies like HTML, CSS, and JavaScript to back-end expertise involving databases and server-side scripting.
It’s a step towards becoming a versatile developer capable of building complete web applications from scratch.
Factorial Program in JavaScript Using for loop (Iterative Approach)
The first method to find factorial in JavaScript is the iterative approach. It includes using a for loop:
Code:
function factorial(n) {
if (n < 0) return "Factorial for negative numbers is not defined";
if (n === 0 || n === 1) return 1;
let result = 1;
for (let i = 2; i <= n; i++) {
result *= i;
}
return result;
}
console.log(factorial(5)); // Testing the function with 5
Output:
120
Explanation:
- The factorial function in JavaScript code above takes one argument n, which is the number whose factorial is to be calculated.
- If n is less than 0, the function returns a message stating that factorial is not defined for negative numbers.
- If n is 0 or 1, the function returns 1, as the factorial of 0 and 1 is 1.
- We initialize a variable result to 1.
- A for loop is set up to run from 2 to n (inclusive). The reason we start from 2 is that multiplying by 1 has no effect.
- In each iteration, we multiply result by the loop variable i. This accumulates the product of all numbers from 2 to n.
- After the loop finishes, result holds the factorial of n, which is then returned.
- Finally, console.log(factorial(5)) is used to test the function and print the factorial of 5, which is 120.
This iterative approach is straightforward and efficient for calculating factorials in JS, especially for smaller values of n.
Upskill Yourself With Live Training
Recommended Courses for You | Book Live Class for FREE! |
Full Stack Web Development Course | Book Now |
WordPress Course | Book Now |
Front-End Development Course | Book Now |
Factorial Program in JavaScript Using Recursion
The second method to find the factorial of a number in JavaScript is the recursive approach. The recursive programming involves a function calling itself to break down the problem into smaller, more manageable parts.
Code:
function factorial(n) {
if (n < 0) return "Factorial for negative numbers is not defined";
if (n === 0 || n === 1) return 1;
return n * factorial(n - 1);
}
console.log(factorial(5)); // Testing the function with 5
Output:
120
Explanation:
- The JavaScript factorial function is defined to take one argument n, the number for which the factorial is to be calculated.
- If n is less than 0, the function returns a message indicating that the factorial is not defined for negative numbers.
- If n is 0 or 1, the function returns 1 since the factorial of 0 and 1 is 1 by definition.
- The function calls itself (factorial(n – 1)) within its return statement. This is the recursive step, where the function keeps calling itself with decremented values of n until it reaches the base case (0 or 1).
- Each call to factorial waits for the result of its recursive call to complete, effectively building a stack of calls. When the base case is reached, these calls resolve in a last-in-first-out order, multiplying the numbers together.
- The product of n * factorial(n – 1) is returned each time until the initial call is resolved, giving us the factorial of the original number.
- The line console.log(factorial(5)) is used to test the function and prints the factorial of 5, which is 120.
This JavaScript program to find factorial of a number is a classic example of how recursion can simplify the code for certain mathematical problems. However, it’s important to note that for very large values of n, this method might lead to a stack overflow error due to too many recursive calls.
Interview Questions for You to Prepare for Jobs
Factorial of Number in JavaScript Using Higher-Order Functions
Higher-order functions in JS are functions that operate on other functions, either by taking them as arguments or by returning them. For calculating the factorial of a number in JavaScript, we can use the Array.prototype.reduce() method, which is a higher-order function.
This approach is a bit more abstract but shows the power of JavaScript’s functional programming capabilities.
Code:
function factorial(n) {
if (n < 0) return "Factorial for negative numbers is not defined";
if (n === 0 || n === 1) return 1;
return Array.from({ length: n }, (_, i) => i + 1)
.reduce((acc, val) => acc * val, 1);
}
console.log(factorial(5)); // Testing the function with 5
Output:
120
Explanation:
- The JS factorial function takes one argument n, the number whose factorial is to be calculated.
- If n is less than 0, the function returns a message stating that factorial is not defined for negative numbers.
- If n is 0 or 1, it returns 1, as the factorial of 0 and 1 is 1.
- Array.from({ length: n }, (_, i) => i + 1) creates an array of numbers from 1 to n. The second argument of Array.from is a map function that fills each array element with its index plus one.
- The reduce() method is used to reduce the array to a single value. It takes a callback function and an initial accumulator value (set to 1).
- The callback function ((acc, val) => acc * val) takes an accumulator (acc) and the current value (val) and multiplies them together. This operation is performed for each element in the array.
- The final result of reduce() is the factorial of n.
This approach using higher-order functions showcases the flexibility and expressive power of JavaScript. It’s particularly useful for those who prefer a more functional programming style. However, it’s important to note that this method might be less intuitive for beginners compared to the iterative or recursive approaches.
Efficiency of JavaScript Factorial Programs
When discussing the efficiency of the three methods (iterative, recursive, higher-order function) for calculating factorials in JavaScript, it’s important to consider both time complexity and space complexity.
1. Iterative Method:
- Time Complexity: O(n) – The iterative approach has a linear time complexity because it involves a single loop that runs n times.
- Space Complexity: O(1) – It uses a constant amount of space, as the number of variables used (like the accumulator for the product) does not increase with input size.
- Efficiency: This method is generally the most efficient in terms of both time and space. It’s straightforward and has a lower overhead because it does not involve function call overhead or the risk of stack overflow.
2. Recursive Method:
- Time Complexity: O(n) – Each call to the recursive function reduces the problem size by 1, leading to n calls in total.
- Space Complexity: O(n) – Recursive approaches have a linear space complexity due to the call stack. Each function call adds a layer to the call stack, which can become significant with large n.
- Efficiency: While recursive methods offer elegant and concise solutions, they are less efficient in terms of space. In JavaScript, particularly, deep recursive calls can lead to stack overflow errors for large values of n.
3. Higher-Order Function Method:
- Time Complexity: O(n) – Similar to the other methods, the higher-order function approach goes through n elements, performing multiplication on each.
- Space Complexity: O(n) – This method creates an array of size n, which adds to the space complexity. The reduce() function itself, however, uses constant space.
- Efficiency: In terms of time, it’s comparable to the other methods, but the space efficiency is lower due to the creation of the array. This method might also have additional overhead due to the creation of function objects and the array manipulation, making it potentially less efficient than the iterative approach.
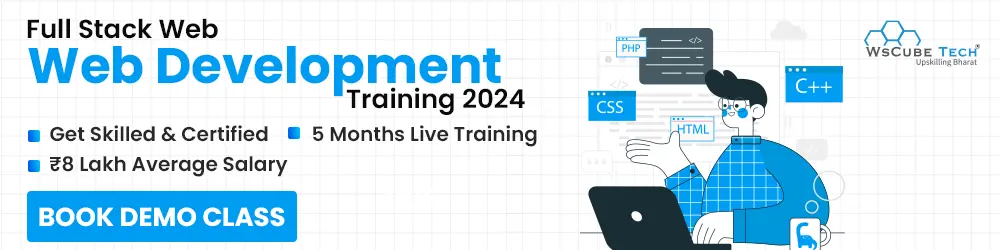
Role of Using JavaScript Factorial Program
The role of using a factorial program in JavaScript extends beyond just solving a mathematical problem; it serves multiple educational and practical purposes, especially for those learning or working in the field of web development.
1. Foundation for Learning Programming Concepts
Factorial programs are excellent for illustrating loop constructs like for, while, and do-while. Beginners can learn how these loops work, including loop initialization, condition checking, and increment/decrement operations.
A factorial program is a classic example of recursion, a fundamental programming concept where a function calls itself.
It also helps in understanding how functions are called and how values are passed and returned in JavaScript.
2. Introduction to Algorithmic Thinking
Factorial program in JS introduces learners to basic algorithmic thinking, as they must figure out the steps to calculate a factorial and then translate these steps into code. It also provides an opportunity to compare different algorithms (iterative vs. recursive) for the same problem.
3. Demonstration of JavaScript Syntax and Features
Writing a factorial program requires understanding and applying JavaScript syntax, including functions, variables, and operators. It’s also a chance to explore JavaScript’s higher-order functions, like reduce(), for more advanced solutions.
4. Error Handling and Input Validation
You can implement error handling and input validation, essential aspects of robust programming, to ensure the program only processes valid numerical inputs.
5. Performance Considerations
It offers an opportunity to discuss performance. For example, recursive solutions, while elegant, may not be as efficient as iterative ones for calculating factorials of larger numbers.
6. Practical Applications in Web Development
While calculating factorials might not be a common task in everyday web development, the skills and concepts learned through this exercise are directly applicable to more complex tasks and projects in JavaScript.
FAQs About Factorial in JavaScript
In mathematics, the factorial of a non-negative integer n is the product of all positive integers less than or equal to n. It’s denoted as n!. For example, the factorial of 5 (5!) is 5 × 4 × 3 × 2 × 1 = 120.
Calculating factorials in JavaScript helps beginners understand key programming concepts such as loops, recursion, and higher-order functions. It also provides a foundational understanding of algorithmic thinking and JavaScript syntax, which are crucial in web development.
The best method depends on the context and your familiarity with JavaScript. The iterative method is straightforward and efficient, the recursive method is elegant but can be less efficient for large numbers, and the higher-order function method demonstrates advanced JavaScript features but might have additional overhead.
Each method has its limitations with very large numbers. The iterative and recursive methods may be more efficient for moderately large numbers, but the recursive method risks stack overflow errors. JavaScript has limitations in handling very large numbers, which might require special handling or libraries.
Yes, the provided programs include basic error handling for negative numbers, as the factorial of a negative number is not defined. Additional checks can be added for data types and other edge cases.
Free Courses for You
Course Name | Course Name |
Django Course | Affiliate Marketing Course |
Semrush Course | Video Editing Course |
Blogging Course | AngularJS Course |
Shopify Course | Photoshop Course |
Wrapping Up:
Mastering the art of calculating factorial in JavaScript is not just about solving a mathematical puzzle—it’s about building a solid foundation in web development and programming concepts.
But why stop there? Build a lucrative career in web development, by joining the live online JavaScript course or the Full Stack Developer course. These courses provide hands-on experience, expert guidance, and the skills needed to thrive in the ever-evolving tech landscape.
Learn more JavaScript programs