JavaScript is the cornerstone language of web development. If you are a beginner or someone working on website development, you must learn various JavaScript programs to show your proficiency and work efficiently.
Among these, the program to find even odd in JavaScript is an interesting and very useful topic. This might look like a simple concept, but it is a practical skill that lays the foundation for more complex coding tasks.
For example, the odd even program in JavaScript is used to alternate row colors in a table or to apply different styles to different elements based on their properties.
So, if you are someone looking to build your career in web development, mastering JavaScript is a critical step. Not only is JavaScript important for front-end development, bringing websites to life, but it’s also increasingly used on the server-side, thanks to Node.js.
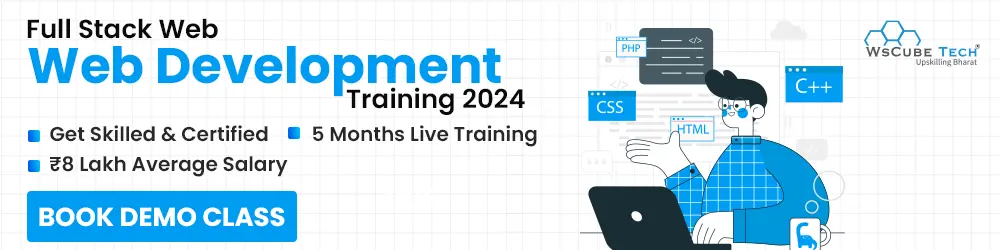
As you begin your upskilling journey, you must go for an expert-led online full-stack developer course. It offers a structured learning path from basic to advanced concepts, including hands-on projects that simulate real-world scenarios.
Now, let’s get started and learn the JavaScript program to find odd or even numbers.
Role of JavaScript Odd or Even Program
Here, we will know the role and uses of the odd or even number program in JS:
- Understanding Basic Programming Concepts: This program introduces beginners to essential programming concepts such as conditional statements (if-else), operators (like the modulo operator %), and basic algorithmic thinking. These are core skills in any programming language, not just JavaScript.
- Grasping JavaScript Syntax and Structure: For those new to JavaScript or programming in general, writing a program to check if a number is odd or even provides an excellent opportunity to get familiar with the syntax and structure of JavaScript. This includes understanding how to declare variables, use functions, and write readable and efficient code.
- Developing Logical Thinking and Problem-Solving Skills: The task requires logical thinking to decide how to approach the problem (using modulo, ternary operator, etc.) and to implement the solution effectively. These problem-solving skills are important in all areas of software development.
- Learning to Debug and Test Code: As simple as it may seem, the program can introduce bugs or unexpected behavior, especially for beginners. Learning to debug and test even such basic code is a crucial web developer skill.
- Foundation for More Complex Operations: This program lays the groundwork for understanding more complex mathematical operations and algorithms. Many advanced concepts in computer science, such as data structures and algorithms, build upon these basic understandings.
- Encouraging Best Practices: Even in simple programs, it’s important to encourage best practices such as code readability, efficiency, and maintainability. Beginners learn to write code that not only works but is also well-structured and easy to understand.
Upskill Yourself With Live Training
Even Odd Program in JavaScript Using Modulo (%) Operator
Following is a simple JavaScript code that uses the modulo operator (%) and function to check if a number is odd or even, along with its output and a brief explanation:
Code:
function checkOddOrEven(number) {
if (number % 2 === 0) {
return "It is Even Number";
} else {
return "It is Odd Number";
}
}
// Get user input from a browser prompt
let userInput = prompt("Enter a number:");
let number = parseInt(userInput, 10); // Convert the input to an integer
console.log(checkOddOrEven(number));
Output:
Enter a number:67
It is Odd Number
Explanation:
- The program defines a function named checkOddOrEven that takes one parameter, number.
- Inside the function, there’s an if statement that uses the modulo operator (%) to check if number is evenly divisible by 2 (number % 2 === 0). This condition is true for even numbers.
- If the condition is true (i.e., the number is even), the function returns the string “It is Even Number”.
- If the condition is not true (i.e., the number is odd), the else block executes, and the function returns the string “It is Odd Number”.
- The program then uses the prompt function to display a dialog box in the web browser, asking the user to “Enter a number”.
- The user’s input is captured and stored in the variable userInput.
- The parseInt function is used to convert the userInput string to an integer. The 10 in parseInt(userInput, 10) specifies that the conversion should be done considering the input as a base-10 (decimal) number.
- The converted integer is stored in the variable number.
- The checkOddOrEven function is called with the user-provided number as its argument.
Odd Even Program in JavaScript Using Ternary Operator
Following is a JavaScript program to check if a number is odd or even using the ternary operator:
Code:
function checkOddOrEven(number) {
return number % 2 === 0 ? "It is Even Number" : "It is Odd Number";
}
// Get user input from a browser prompt
let userInput = prompt("Enter a number:");
let number = parseInt(userInput, 10); // Convert the input to an integer
console.log(checkOddOrEven(number));
Output:
Enter a number:32
It is Even Number
Explanation:
- The function checkOddOrEven takes a number as input and uses the ternary operator (? 🙂 to return “It is Even Number” if the number is divisible by 2 without a remainder, and “It is Odd Number” otherwise.
- A prompt is introduced to capture user input. The prompt(“Enter a number:”) displays a dialog box asking the user to enter a number.
- The input received from the user is a string. The parseInt(userInput, 10) function is used to convert this string into an integer. The second argument, 10, specifies that the input should be parsed as a base-10 (decimal) number.
Odd or Even Program in JavaScript Using Bitwise & Operator
Using the bitwise AND (&) operator is a more advanced and less commonly used method for checking if a number is odd or even in JavaScript.
Code:
function checkOddOrEven(number) {
return (number & 1) ? "It is Odd Number" : "It is Even Number";
}
console.log(checkOddOrEven(7));
Output:
It is Odd Number
Explanation:
- The function checkOddOrEven takes a single argument, number.
- Inside the function, the bitwise AND operator (&) is used. This operator compares each bit of the number in its binary form to the bits of 1 (which is 0001 in binary).
- The expression (number & 1) will be 1 (or true) if the least significant bit of number is 1, indicating an odd number. If the least significant bit is 0, the expression will be 0 (or false), indicating an even number.
- The ternary operator is then used: if (number & 1) is true (the number is odd), it returns “It is Odd Number”; otherwise, it returns “It is Even Number”.
This bitwise method is a more low-level approach and can be faster than using the modulo operator, as it directly operates on the binary representation of the number. However, it’s less intuitive, especially for beginners, and is generally used in scenarios where performance is a critical concern.
Interview Questions for You to Prepare for Jobs
Odd Even Program in JavaScript Using Arrays
Using arrays to check if a number is odd or even in JavaScript is an unconventional but interesting approach.
Code:
function checkOddOrEven(number) {
const results = ['Even', 'Odd'];
return results[number % 2];
}
console.log(checkOddOrEven(15));
Output:
Odd
Explanation:
- The function checkOddOrEven takes a single argument, number.
- Inside the function, an array results is declared with two elements: “Even” and “Odd”. These elements correspond to the indices 0 and 1 in the array, respectively.
- The function then returns an element from the results array based on the result of number % 2. The modulo operation will yield 0 for even numbers and 1 for odd numbers.
- The expression number % 2 serves as the index to access the elements of the results array.
- If number is even, number % 2 will be 0, and results[0] (which is “Even”) will be returned. If number is odd, number % 2 will be 1, and results[1] (which is “Odd”) will be returned.
- An example is provided using console.log. checkOddOrEven(15) returns “Odd” because 15 is an odd number.
This method of using arrays to check if a number is odd or even in JS is a creative way to show array indexing and the use of the modulo operator. It’s a clear example of how different programming concepts can be combined in JavaScript to achieve a specific outcome.
JavaScript Odd Even Program Using for Loop
Using a for loop to check if a number is odd or even in JavaScript is not a conventional approach and is generally less efficient than using direct calculations like the modulo operator.
Code:
function checkOddOrEven(number) {
let isEven = true;
for (let i = 0; i < number; i++) {
isEven = !isEven;
}
return isEven ? "Even" : "Odd";
}
console.log(checkOddOrEven(9));
Output:
Odd
Explanation:
- The function checkOddOrEven takes one argument, number.
- Inside the function, a boolean variable isEven is initialized to true.
- A for loop is set up to run from 0 to number – 1. With each iteration, the value of isEven is toggled (if it’s true, it becomes false, and vice versa).
- For an even number, the loop will toggle isEven an even number of times, ending up back at true. For an odd number, the loop will toggle isEven an odd number of times, ending up at false.
- After the loop, the ternary operator checks isEven. If isEven is true, “Even” is returned; otherwise, “Odd” is returned.
This method is more of a conceptual or experimental approach to understanding loops and boolean toggling. It’s less practical for real-world applications due to its inefficiency, especially for large numbers, but serves as a good learning tool for beginners in JavaScript.
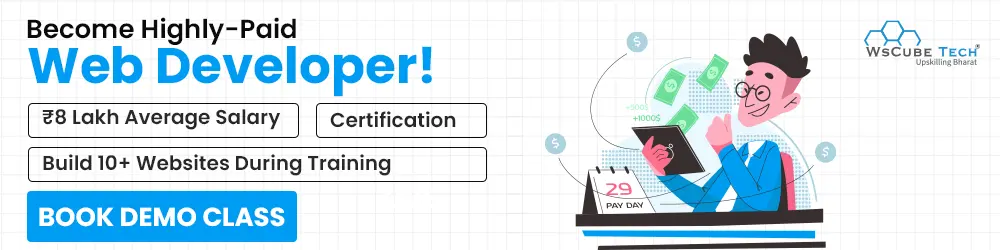
FAQs About Even Odd Number in JavaScript
The simplest and most common method is using the modulo operator (%). If a number modulo 2 equals 0, it’s even; otherwise, it’s odd.
While bitwise operators like & can be efficient in terms of computation, their usage for this particular task is less intuitive. They are generally used in more complex scenarios that involve low-level bit manipulation.
These functions are designed for integers. If you pass a decimal number, it will first be converted to an integer (truncated) before performing the odd/even check.
Using a for loop is more of an educational exercise to understand looping and boolean toggling. It’s not a practical approach in real-world applications due to its inefficiency, especially for large numbers.
JavaScript does not have a built-in function specifically for checking if a number is odd or even. However, you can easily create a custom function using the modulo operator or other methods demonstrated in this blog.
Yes, the methods described in this blog post are based on fundamental JavaScript operations and can be used in any environment where JavaScript runs, such as browsers and Node.js.
Free Courses for You
Wrapping Up:
In this tutorial, we went through various methods to check if a number is odd or even in JavaScript.
To dive deeper and truly use the power of JavaScript, consider enrolling in the live online JavaScript course or the comprehensive full stack developer course. These courses offer not just in-depth knowledge but also practical, real-world skills essential for upskilling and building a thriving career in tech.
Learn more JavaScript programs