Prime numbers are the unique integers greater than 1 that have no divisors other than 1 and themselves. These numbers have been a subject of interest and study for centuries. Their distinct properties captivate mathematicians and play a crucial role in areas like cryptography, making them a crucial concept in modern technology.
As we learn how to identify these mathematical numbers, we turn to JavaScript, a dynamic and versatile language that stands at the heart of web development. JavaScript is an essential language in web development, shaping interactions, animations, and the overall user experience.
By learning to check a prime number in JavaScript, you will sharpen your skills in a language that powers millions of websites worldwide.
Remember, the JavaScript prime number program is more than a coding exercise; it’s a step towards developing a computational thinking mindset, essential for solving complex problems.
And if you’re aspiring to learn web development, consider going for the best Full Stack Developer course. It is designed to guide you through both front-end and back-end technologies, turning you into a high-paying developer capable of handling diverse challenges.
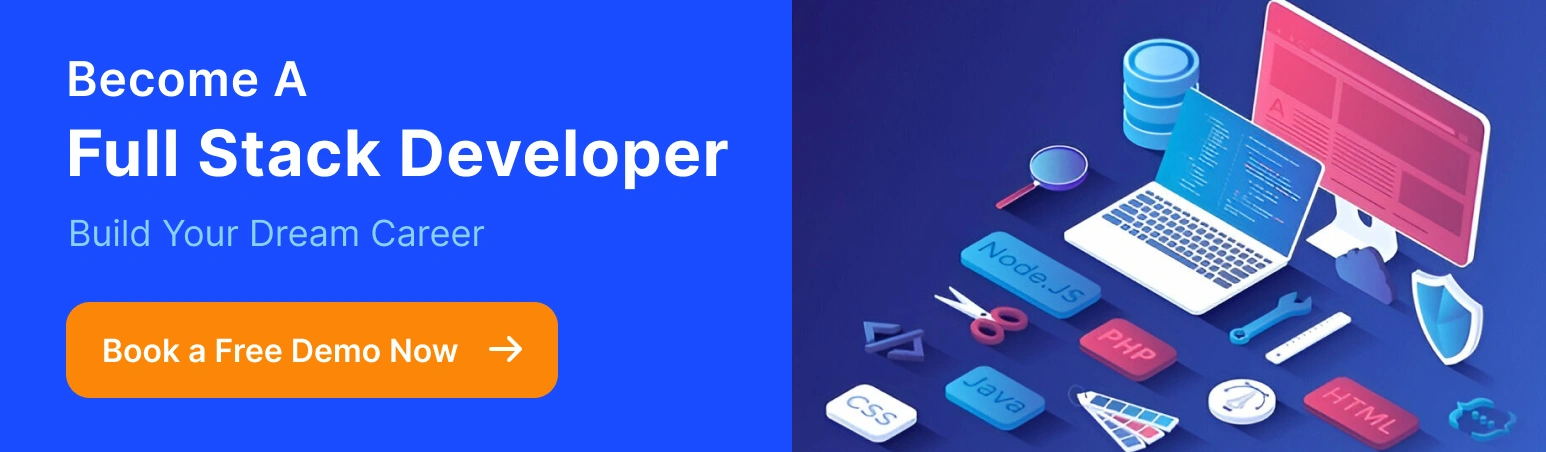
Role of JavaScript Prime Number
The role of using a program for prime number in JS extends beyond just identifying whether a number is prime. This task serves several important functions, particularly for beginner programmers and those interested in web development:
1. Understanding Fundamental Programming Concepts
- Looping Structures: Learning how to check for prime numbers in JavaScript introduces essential looping concepts like for and while loops.
- Conditionals: The task involves making decisions using if-else statements, a fundamental concept in programming.
- Boolean Logic: Understanding how to return true or false based on a number’s primality is a practical application of boolean logic.
2. Developing Algorithmic Thinking
- Problem Solving: Checking for prime numbers in JS challenges beginners to think algorithmically, breaking down a problem into smaller steps.
- Efficiency Awareness: By trying different methods (basic to advanced), you understand the importance of computational efficiency.
3. Practicing Debugging and Error Handling
- Debugging: As a beginner, you learn to debug by addressing common issues like incorrect loop bounds or data type mismatches.
- Error Handling: Implementing input validation teaches handling unexpected or incorrect user input.
4. Enhancing JavaScript Skills for Web Development
- Function Creation: Encapsulating the logic into functions fosters reusable code practices, a key skill in JavaScript. Go for an online JavaScript course to upskill with experienced mentors.
- Interactive Web Elements: Integrating this functionality into a web page, such as checking user-inputted numbers, shows how JavaScript interacts with HTML/CSS.
5. Foundation for Advanced Topics
- Algorithm Complexity: Leads to discussions on time complexity and Big O notation.
- Data Structures and Algorithms: Prepares for more complex algorithms and data structure understanding.
6. Career Relevance
- Job Interviews: Common in coding interviews to test basic programming skills.
- Real-world Applications: The principles learned are applicable in various programming tasks in website development.
Prime Number Program in JavaScript Using Function
To check for a prime number in JavaScript using a for loop with user input, we can write a simple function and then call it with a number provided by the user. This is the simplest approach to understanding prime number validation.
JavaScript Code:
function isPrime(num) {
if (num <= 1) return false; // Numbers less than or equal to 1 are not prime
for (let i = 2; i < num; i++) {
if (num % i === 0) {
return false; // If num is divisible by any number other than 1 and itself
}
}
return true; // If no divisors were found, num is a prime number
}
// Example of user input (in a real-world scenario, this could be from a form or console input)
const userInput = 11;
console.log(`Is ${userInput} a prime number? `, isPrime(userInput));
Output:
Is 11 a prime number? true
Explanation:
The isPrime function checks if a number is prime.
The function first checks if the number is less than or equal to 1. Numbers less than or equal to 1 are not considered prime, so it immediately returns false in such cases.
The for loop starts from 2 (the first prime number) and continues until it reaches one less than the number being checked. For each iteration, it checks if the num is divisible by i (num % i === 0). If it finds a divisor, it returns false, indicating that num is not a prime number.
If the loop completes without finding any divisors, the function returns true, indicating that the number is prime.
JavaScript Prime Number Program (Efficient Way)
While the above program is the simplest approach, we will now learn the most efficient approach as well, in terms of computation resources used in the program.
Computational efficiency means how effectively a program uses time and memory resources to produce a result. In programming, efficient code means faster execution and less usage of computing resources, which is crucial for handling large datasets or performing complex calculations.
Efficiency becomes particularly important when dealing with algorithms, where the choice of algorithm and how it’s implemented can significantly impact performance. Inefficient algorithms can lead to slow execution times and high resource consumption, which is a critical concern in real-world applications.
Let’s take the example of checking for a prime number in JavaScript, which includes iterating through all numbers from 2 to num – 1 to check for divisors.
JavaScript Code:
function isPrime(n) {
// Check if n=1 or n=0
if (n <= 1)
return false;
// Check if n=2 or n=3
if (n == 2 || n == 3)
return true;
// Check whether n is divisible by 2 or 3
if (n % 2 == 0 || n % 3 == 0)
return false;
// Check from 5 to square root of n
// Iterate i by (i+6)
for (var i = 5; i <= Math.sqrt(n); i = i + 6)
if (n % i == 0 || n % (i + 2) == 0)
return false;
return true;
}
// Get user input from a browser prompt
let userInput = prompt("Enter a number:");
let number = parseInt(userInput, 10); // Convert the input to an integer
// Check if the number is prime and display an appropriate message
isPrime(number) ? console.log(`${number} is a prime number.`) : console.log(`${number} is not a prime number.`);
Output:
Enter a number:74
74 is not a prime number.
Explanation:
- The isPrime function is optimized to check if a number is prime. It efficiently handles edge cases and checks divisibility, improving performance by only iterating up to the square root of n.
- The program uses prompt to ask the user to enter a number.
- The entered value, which is a string, is converted to an integer using parseInt.
- The program then checks if the entered number is prime using the isPrime function.
- Depending on the result, it prints either “[number] is a prime number.” or “[number] is not a prime number.”, with [number] being the user-entered number.
JS Prime Number Program Using Sieve of Eratosthenes
The Sieve of Eratosthenes is a highly efficient way to find all prime numbers up to a large number and is a great example of an algorithm that uses a simple idea to solve a problem more efficiently than checking each number individually.
Below is an example to find all prime numbers up to 20.
JavaScript Code:
function sieveOfEratosthenes(limit) {
let primes = [];
let sieve = Array(limit + 1).fill(true);
sieve[0] = sieve[1] = false; // 0 and 1 are not prime numbers
for (let i = 2; i <= Math.sqrt(limit); i++) {
if (sieve[i]) {
for (let j = i * i; j <= limit; j += i) {
sieve[j] = false; // Marking multiples of i as non-prime
}
}
}
// Collecting all prime numbers
for (let i = 2; i <= limit; i++) {
if (sieve[i]) primes.push(i);
}
return primes;
}
console.log(sieveOfEratosthenes(20)); // Example usage
Output:
[2, 3, 5, 7, 11, 13, 17, 19]
Explanation:
- The sieveOfEratosthenes function is designed to list all prime numbers up to a given limit.
- An array sieve is created and initialized to true to indicate that all numbers are initially considered prime.
- sieve[0] and sieve[1] are set to false since 0 and 1 are not prime numbers.
- The outer for loop iterates from 2 to the square root of the limit. The square root boundary is used for optimization, as any non-prime number must have a factor less than or equal to its square root.
- If a number i is marked true (prime), the inner for loop marks all multiples of i starting from i * i as false (non-prime). We start from i * i because smaller multiples of i would have been marked by smaller primes.
- Another loop is used to traverse the sieve array. If an element at index i is true, i is a prime number and is added to the primes array.
JavaScript Program for Prime Numbers in Range
To check for prime numbers within a specific range, we can create a JavaScript function that iterates through the range, checks each number for primality, and collects the prime numbers.
Below is an example to find prime numbers between 100 to 200.
JavaScript Code:
function findPrimesInRange(start, end) {
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
}
return true;
}
let primes = [];
for (let i = start; i <= end; i++) {
if (isPrime(i)) {
primes.push(i);
}
}
return primes;
}
const start = 100;
const end = 200;
const primeNumbers = findPrimesInRange(start, end);
console.log(`${primeNumbers.join(', ')} are prime numbers`);
Output:
101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199 are prime numbers
Explanation:
- The isPrime function checks whether a given number is prime. It returns true if the number is prime and false otherwise.
- The findPrimesInRange function iterates over all numbers from start to end (inclusive).
- For each number, it calls the isPrime function to check if it is prime.
- If the number is prime, it is added to the primes array.
- After collecting all the prime numbers in the range, they are joined into a string separated by commas using join(‘, ‘).
JavaScript Prime Numbers From 1 to 100
Now, let’s write a JavaScript program to check prime numbers from 1 to 100. The program will use a function to check if a number is prime and then iterate from 1 to 100, printing each prime number it finds.
JavaScript Code:
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
}
return true;
}
function printPrimes(limit) {
let primes = [];
for (let i = 1; i <= limit; i++) {
if (isPrime(i)) {
primes.push(i);
}
}
console.log(`Prime numbers from 1 to ${limit}: ${primes.join(', ')}`);
}
printPrimes(100); // Print prime numbers from 1 to 100
Output:
Prime numbers from 1 to 100: 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97
Explanation:
- The isPrimefunction checks if a given number num is prime.
- It returns false for numbers less than or equal to 1.
- For other numbers, it checks for divisors up to the square root of num. If a divisor is found, it returns false.
- This function iterates from 1 to the specified limit (100 in this case).
- For each number, it uses the isPrime function to check if the number is prime.
- If the number is prime, it’s added to the primes array.
- The prime numbers are joined into a string using join(‘, ‘) and printed to the console with a message.
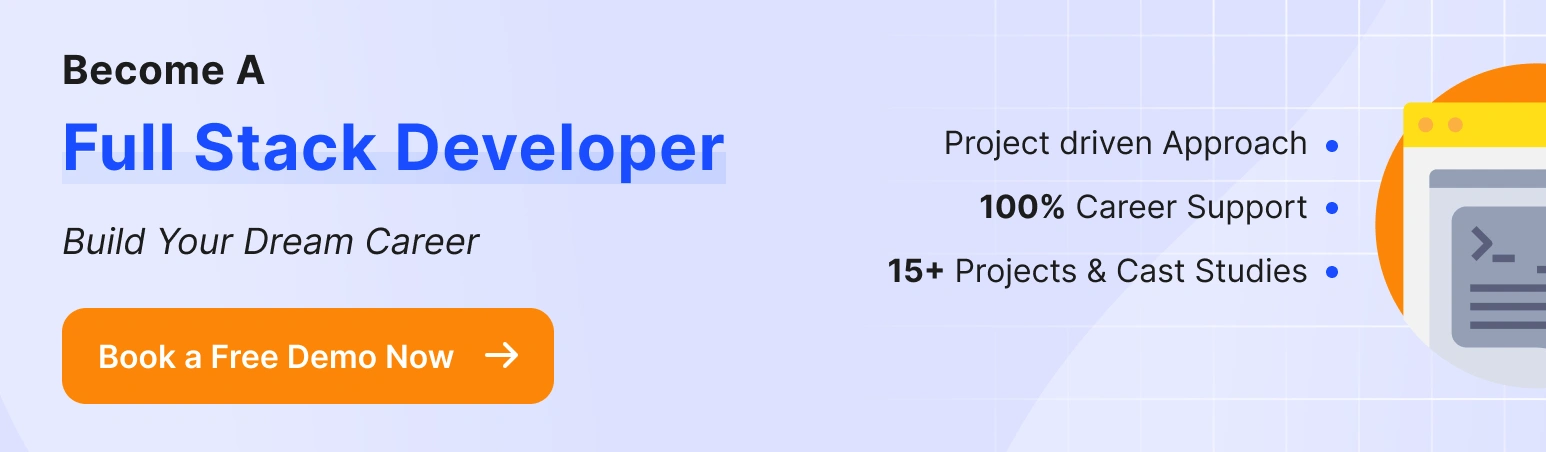
FAQs About JavaScript Prime Number
In JavaScript, a prime number is the same as in mathematics: a number greater than 1 that has no positive divisors other than 1 and itself. For example, 2, 3, 5, and 7 are prime numbers.
JavaScript doesn’t have a built-in method to determine prime numbers. Instead, you can write a function that checks if a number is divisible only by 1 and itself. This usually involves using a loop to test divisibility by all smaller numbers.
JavaScript can handle large numbers, but the efficiency depends on the algorithm used. For very large numbers, specialized algorithms and optimizations are required for efficient prime number checking.
JavaScript can accurately represent integers up to 2^53 – 1 (Number.MAX_SAFE_INTEGER). For numbers larger than this, there’s a risk of precision loss, making reliable prime checks challenging.
You can optimize the function by checking for divisibility only up to the square root of the number, and by skipping even numbers after checking for 2.
By definition, prime numbers are positive. If your function receives a negative number, it should return false, as negative numbers cannot be prime.
While JavaScript doesn’t have a standard library for prime numbers, there are third-party libraries and npm packages that provide functionality for prime number checking and related mathematical operations.
Yes, a JavaScript function to check for prime numbers can be used in both browser environments (like Chrome, Firefox) and server environments (like Node.js), as long as it doesn’t rely on environment-specific features.
You can handle errors by checking the input type and range before processing and using try…catch blocks to manage exceptions in more complex functions.
Yes, you can write a JavaScript function that iterates through a range of numbers and uses your prime checking function to build and return a list of prime numbers within that range.
Wrapping Up:
Learning how to check prime number in JS is just the beginning of your journey in coding. To further upgrade your skills and open new doors in your career, book a live class of online JavaScript course or a comprehensive full-stack developer course. These courses offer in-depth knowledge, practical experience, and the opportunity to stay ahead in today’s competitive job market.
Learn more JavaScript programs