In our series of JavaScript programs, today, we’re going to learn how to print Fibonacci series in JavaScript.
Also called the Fibonacci Sequence, it is a series of numbers where each number is the sum of the two preceding ones. It is not just a mathematical concept; but also important for algorithms and programming logic.
JavaScript stands as a pillar in web development. It’s a language that breathes life into websites, turning static pages into interactive experiences. Understanding how to manipulate numbers and algorithms in JavaScript is crucial for anyone looking to excel in this field, especially for those aspiring to become full-stack developers.
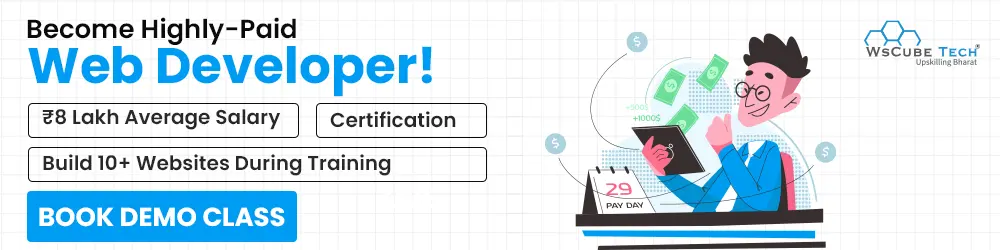
Speaking of which, if you’re aiming to build your career in web development, book live demo class of the online Full Stack Developer course.
What is Fibonacci Series?
The Fibonacci series is a sequence of numbers in which each number (after the first two) is the sum of the two preceding ones. The sequence starts with 0 and 1.
Here’s a simple example to understand the Fibonacci series:
- Start with two numbers: 0 and 1.
- Add them together: 0 + 1 = 1. So, the third number in the sequence is 1.
- Continue this pattern: Add the last two numbers to get the next one.
Fibonacci Series in JavaScript Using for loop
Here’s an example of Fibonacci code in JavaScript using a for loop:
JavaScript Code:
function fibonacciSeries(n) {
let fib = [0, 1];
for (let i = 2; i < n; i++) {
fib[i] = fib[i - 1] + fib[i - 2];
}
return fib.slice(0, n);
}
// Example: Generate the first 10 numbers in the Fibonacci series
console.log(fibonacciSeries(10));
Output:
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Explanation:
- The function fibonacciSeries(n) is defined to calculate the Fibonacci series up to n numbers.
- We start with an array fib initialized with the first two numbers of the Fibonacci series [0, 1].
- The for loop starts from index 2 and runs until it reaches the length n specified by the user. This is because the first two numbers of the series are already defined.
- Inside the loop, each number is calculated by adding the two preceding numbers in the series. This is done using fib[i – 1] + fib[i – 2] and the result is stored in fib[i].
- The function returns the Fibonacci series up to n numbers using fib.slice(0, n). The slice method is used to ensure that the length of the returned array matches the number n specified by the user.
- Finally, we call fibonacciSeries(10) to print the first 10 numbers of the Fibonacci series to the console.
This code is a simple and efficient way to use JavaScript for Fibonacci series and is particularly useful for beginners to understand how arrays and loops work in the language.
Upskill Yourself With Live Training
Recommended Courses for You | Book Live Class for FREE! |
Full Stack Web Development Course | Book Now |
WordPress Course | Book Now |
Front-End Development Course | Book Now |
Fibonacci Sequence in JavaScript Using Recursion
Below is an example of generating the Fibonacci number in JavaScript using recursion:
JavaScript Code:
function fibonacci(n) {
if (n < 2) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Example: Print the 10th number in the Fibonacci series
console.log(fibonacci(10));
Output:
55
Explanation:
- The function fibonacci(n) is defined to calculate the nth number in the JS Fibonacci series using recursion.
- The if statement if (n < 2) { return n; } serves as the base case. For n less than 2 (i.e., 0 or 1), the function simply returns n, as the first two numbers of the Fibonacci series are 0 and 1, respectively.
- The function makes two recursive calls: fibonacci(n – 1) and fibonacci(n – 2). This represents the definition of the Fibonacci series, where each number is the sum of the two preceding ones.
- The function returns the sum of these two recursive calls. As the recursion unfolds, it eventually reaches the base case, which provides the values to be summed up.
- The line console.log(fibonacci(10)); prints the 10th number in the Fibonacci series, which is 55.
Recursion is a powerful concept in programming, and this example shows how a complex problem like generating the Fibonacci series in JS can be solved easily.
However, it’s important to note that recursive solutions can be less efficient for large values of n due to the increased number of function calls and the risk of stack overflow. For such cases, iterative solutions or dynamic programming approaches are generally preferred.
Fibonacci Series in JavaScript Using Array
The third method is to generate the Fibonacci series in JavaScript using an array:
JavaScript Code:
function fibonacciSeries(n) {
if (n === 1) return [0];
if (n === 2) return [0, 1];
const fib = [0, 1];
for (let i = 2; i < n; i++) {
fib.push(fib[i - 1] + fib[i - 2]);
}
return fib;
}
// Example: Generate the first 10 numbers in the Fibonacci series
console.log(fibonacciSeries(10));
Output:
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Explanation:
- The function fibonacciSeries(n) is designed to generate the first n numbers of the Fibonacci series.
- The initial if statements handle special cases: if n is 1, it returns an array with only the first element [0], and if n is 2, it returns the first two elements [0, 1].
- The array fib is initialized with the first two elements of the Fibonacci series, [0, 1].
- A for loop is used to iterate from the third element (index 2) up to the nth element.
- Within the loop, each new element is generated by adding the two preceding elements to the array. This is achieved with fib.push(fib[i – 1] + fib[i – 2]).
- The function returns the array fib, which contains the first n numbers of the Fibonacci series.
- Finally, fibonacciSeries(10) is called to print the first 10 numbers of the Fibonacci series.
This approach of using an array to generate the Fibonacci series in JavaScript is efficient and straightforward. It allows beginners to understand how to manipulate arrays and use loops effectively in JavaScript.
Fibonacci Series in JavaScript Using while loop
The following is an example of how to generate Fibonacci series in JavaScript using a while loop:
JavaScript Code:
function fibonacciSeries(n) {
let fib = [0, 1];
let i = 2;
while (i < n) {
fib[i] = fib[i - 1] + fib[i - 2];
i++;
}
return fib.slice(0, n);
}
// Example: Generate the first 10 numbers in the Fibonacci series
console.log(fibonacciSeries(10));
Output:
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Explanation:
- The fibonacciSeries(n) function is designed to calculate the Fibonacci series up to n numbers.
- The Fibonacci series is initialized with the first two numbers, [0, 1].
- The while loop starts with i = 2 and continues as long as i < n. This is because the first two elements of the series are already set.
- Inside the loop, each new Fibonacci number is calculated by summing the two previous numbers in the array: fib[i] = fib[i – 1] + fib[i – 2].
- After each iteration, i is incremented by 1.
- The function returns the array fib containing the Fibonacci series up to n numbers, using fib.slice(0, n) to ensure the series length matches n.
- When the function is called with fibonacciSeries(10), it prints the first 10 numbers of the Fibonacci series.
Using a while loop for generating the Fibonacci series in JavaScript is an effective way to understand loop control and array manipulation. It offers a different perspective from the traditional for loop method and is equally efficient for such tasks.
Interview Questions for You to Prepare for Jobs
Fibonacci Series in JavaScript Using Generator Function (ES6)
Generating the Fibonacci sequence in JS using a generator function is an advanced approach that uses ES6 features.
JavaScript Code:
function* fibonacciGenerator(n) {
let [a, b] = [0, 1];
while (n-- > 0) {
yield a;
[a, b] = [b, a + b];
}
}
// Example: Generate the first 10 numbers in the Fibonacci series
const gen = fibonacciGenerator(10);
let result = [];
for (let value of gen) {
result.push(value);
}
console.log(result);
Output:
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Explanation:
- The fibonacciGenerator(n) function is a generator function, as denoted by the function* syntax. It is designed to yield each number in the Fibonacci series up to n numbers.
- The variables a and b are initialized to the first two numbers of the Fibonacci series, 0 and 1.
- The while loop runs until n reaches 0. Inside the loop, yield a is used to output the current value of a.
- After yielding a, the values of a and b are updated to the next two numbers in the series using array destructuring: [a, b] = [b, a + b].
- Outside the function, the generator fibonacciGenerator(10) is initialized. A for…of loop is used to iterate over the generator, and the yielded values are pushed into the result array.
- Finally, console.log(result) prints the first 10 numbers of the Fibonacci series.
Generator functions are powerful in JavaScript for handling sequences and iterators. They allow you to define an iterative algorithm by writing a single function whose execution is not continuous. In this case, it provides an elegant way to generate the Fibonacci series, yielding one number at a time as needed.
Fibonacci Series Program in JavaScript (User Input)
To create a JavaScript program that generates the Fibonacci series up to a specified number based on user input, we can modify the Fibonacci series function to accept the user’s input and then continue to generate numbers in the series until the next number would be greater than the specified number.
JavaScript Code:
function fibonacciSeriesUpTo(maxValue) {
if (maxValue === 0) return [0];
if (maxValue === 1) return [0, 1];
let fib = [0, 1];
while (true) {
let nextFib = fib[fib.length - 1] + fib[fib.length - 2];
if (nextFib > maxValue) break;
fib.push(nextFib);
}
return fib;
}
// Prompt user for input
let userInput = prompt("Enter the number:");
userInput = parseInt(userInput, 10); // Convert the input to an integer
if (!isNaN(userInput)) {
console.log(fibonacciSeriesUpTo(userInput));
} else {
console.log("Please enter a valid number.");
}
Output:
Enter the number:2000
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]
Role of Using Fibonacci Series Program in JavaScript
- Understanding Fundamental Programming Concepts: The Fibonacci sequence is an excellent tool for understanding basic programming concepts such as loops (for, while), recursion, and conditionals (if-else statements). It provides a clear and tangible example of how these concepts are implemented in code.
- Practicing Algorithmic Thinking: Creating a program to generate the Fibonacci sequence in JavaScript helps in developing algorithmic thinking. It encourages learners to break down a problem into smaller parts, understand the sequence of operations, and implement a solution effectively.
- Introduction to JavaScript Syntax: For beginners, it’s crucial to get comfortable with the syntax of a programming language. The Fibonacci sequence exercise allows learners to familiarize themselves with JavaScript syntax, functions, and best practices in a structured way.
- Demonstrating Recursion: The Fibonacci sequence is used to introduce the concept of recursion in programming. Recursion is a fundamental concept in computer science and understanding it is essential for more advanced programming topics.
- Enhancing Problem-Solving Skills: Developing a Fibonacci series program involves logical thinking and problem-solving skills. It challenges learners to think critically about how to approach a problem and find efficient solutions.
- Real-World Application: The Fibonacci sequence, while a mathematical concept, has applications in computer algorithms, financial models, and even in nature and art. Understanding how to program this sequence provides insights into how mathematical concepts are applied in various fields.
- Understanding Performance Implications: Implementing the Fibonacci sequence in different ways (e.g., iterative vs. recursive) allows learners to understand the performance implications of different coding approaches, such as time complexity and memory usage.
- Building a Foundation for Advanced Topics: As learners become comfortable with basic programming tasks like the Fibonacci sequence, they lay the groundwork for tackling more complex programming challenges, which is essential for a full-stack developer.
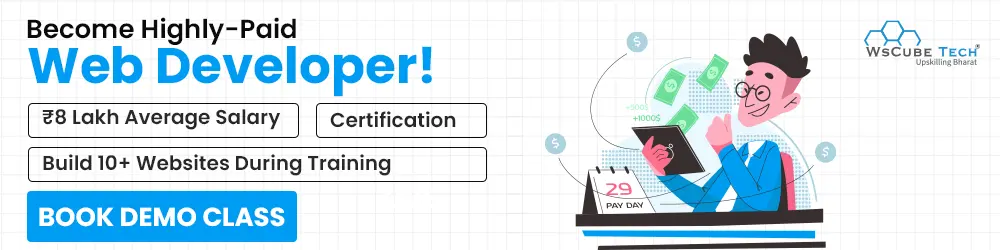
FAQs About Fibonacci Sequence in JavaScript
Learning to program the Fibonacci Sequence in JavaScript helps beginners understand fundamental programming concepts like loops, recursion, and array manipulation. It’s a practical way to get hands-on experience with algorithmic thinking and coding syntax in JavaScript.
Yes, the Fibonacci Sequence can be generated using both iterative methods (like for loops and while loops) and recursive methods in JavaScript. Each method offers different insights into problem-solving and code efficiency.
Both recursion and iteration have their pros and cons. Recursion is more elegant and easier to understand but can be less efficient and more memory-intensive for large sequences. Iteration, on the other hand, is generally more efficient and faster, especially for large sequences.
The Fibonacci Sequence is used in various real-world applications, including algorithm optimization, financial planning, and even in the analysis of biological structures and phenomena.
Learning to code the Fibonacci Sequence in JavaScript is a step towards mastering fundamental programming skills, which are crucial for a web developer. It helps build a strong foundation in front-end technologies and enhances problem-solving skills, both of which are essential in full-stack development.
Yes, especially with recursive methods for large Fibonacci numbers, as it can lead to a significant number of function calls and, consequently, a stack overflow error. Iterative methods are generally more efficient in terms of performance.
Absolutely! JavaScript can be used to generate and display the Fibonacci Sequence on a web page. You can use document manipulation methods to create and insert elements into the HTML to display the sequence.
Free Courses for You
Course Name | Course Name |
Django Course | Affiliate Marketing Course |
Semrush Course | Video Editing Course |
Blogging Course | AngularJS Course |
Shopify Course | Photoshop Course |
Wrapping Up:
As we’ve learned the Fibonacci sequence in JavaScript, it’s clear that such exercises are more than just coding challenges; they’re stepping stones in your journey as a developer.
To further refine your skills and take a leap in your career, book the live demo class of online JavaScript course or a full-stack developer course by WsCube Tech. These courses offer structured learning, real-world projects, and expert guidance, making you a skilled professional.