Today, we’re diving into an essential skill every budding web developer should master: How to reverse a string in JavaScript.
This might seem like a simple task at first glance, but it’s a fundamental concept that lays the groundwork for more complex programming challenges you’ll encounter on your journey to becoming a web developer.
Understanding how to manipulate strings, or sequences of characters, is crucial in web development. Whether you’re working on form validations, data processing, or simply want to display information in a new way, being able to reverse a string in JavaScript can come in handy.
It’s not just about the action itself but learning the thought process and techniques that can be applied to a wide range of problems.
For those who are new to programming or looking to strengthen their web development skills, this post is designed with you in mind.
And if you’re serious about taking your web development skills to the next level, book the live demo class of the online full-stack developer course by WsCube Tech. It is a great way to build a strong foundation in web development, including mastering JavaScript and much more.
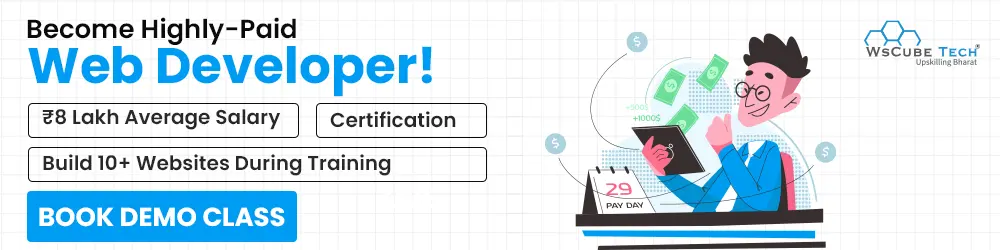
Reverse a String in JavaScript Using Inbuilt Function
Let’s write a JavaScript program to reverse a string using built-in methods. It is a straightforward process that includes three main steps: splitting the string into an array of characters, reversing the array, and then joining the array back into a string.
JavaScript Code:
function reverseString(str) {
return str.split("").reverse().join("");
}
console.log(reverseString("Welcome to WsCube Tech!"));
Output:
!hceT ebuCsW ot emocleW
Explanation:
- str.split(“”): This method splits the string str into an array of individual characters. The “” (empty string) as an argument tells the method to split the string at every character.
- .reverse(): This method reverses the array in place. The first array element becomes the last, and the last array element becomes the first. Since strings in JavaScript are immutable (meaning they cannot be changed once created), we first need to convert the string into an array to apply this method.
- .join(“”): After reversing the array in JS, this method joins all elements of the array back into a string. Similar to split, the “” (empty string) as an argument tells the method to join the array elements without any characters in between them.
By chaining these methods together, we can efficiently reverse a string in JavaScript in a single line of code.
Upskill Yourself With Live Training (Book Free Class)
Reverse String in JavaScript Using for Loop
Here, we will reverse a string in JavaScript using a for loop. It involves iterating over the string from the end to the beginning and constructing a new string by appending each character in reverse order.
This method provides a good opportunity to understand loop control structures and string manipulation without relying on built-in methods like split, reverse, and join.
JavaScript Code:
function reverseString(str) {
let reversed = "";
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
console.log(reverseString("Upskill With WsCube Tech"));
Output:
hceT ebuCsW htiW llikspU
Explanation:
Initialization: A new variable reversed is initialized as an empty string. This will eventually hold the reversed string.
For Loop: The loop is set to start from the last character of the input string (str.length – 1) and decrement the index i until it reaches 0, the index of the first character of the string.
- i = str.length – 1: Initializes i to the index of the last character in the string.
- i >= 0: Continues the loop as long as i is greater than or equal to 0.
- i–: Decreases i by 1 after each iteration, moving backwards through the string.
String Concatenation: In each iteration of the loop, the character at the current index i of the string str is concatenated to the reversed string. This builds up the reversed string in reverse order of the original string.
Return: After the loop completes, the reversed string, which now contains the original string in reverse order, is returned.
Using a for loop to reverse a string in JS is a fundamental approach that highlights the importance of understanding loops and string manipulation. It’s especially useful in environments or situations where you might need to avoid using built-in methods for performance reasons or to meet specific coding standards.
Also Read: 25 Best Web Development Projects in 2024 [With Source Code]
JavaScript Reverse String Using Recursion
Reverse of a string in JavaScript using recursion involves creating a function that calls itself with a progressively smaller piece of the string, building up the reversed string as the recursion unwinds. This method shows the power and elegance of recursion in solving problems that can be broken down into smaller, similar problems.
JavaScript Code:
function reverseString(str) {
// Base case: if the string is empty or has only one character, return the string
if (str.length <= 1) {
return str;
}
// Recursive case: return the last character + reverseString of all characters except the last
return str.charAt(str.length - 1) + reverseString(str.substring(0, str.length - 1));
}
console.log(reverseString("Welcome to WsCube Tech"));
Output
hceT ebuCsW ot emocleW
Explanation:
Base Case: The recursion has a base case that checks if the string str is either empty (str.length === 0) or contains a single character (str.length === 1). In either case, the string cannot be further divided and is returned as is. This condition prevents infinite recursion and provides a clear stopping point.
Recursive Case: For strings longer than one character, the function:
- Takes the last character of the string (str.charAt(str.length – 1)) and appends it to the result of the recursive call.
- The recursive call is made on the substring that excludes the last character (str.substring(0, str.length – 1)). This effectively reduces the problem size with each call, moving closer to the base case.
Combining Results: As the recursion unwinds (i.e., as each call returns to its caller), the last character of each substring is appended in reverse order, constructing the reversed string piece by piece.
Reverse Of String in JavaScript Using Spread Operator (ES6)
The JavaScript program to reverse a string using the spread operator is an elegant and concise way to convert the string into an array of characters, reverse the array, and then join it back into a string.
The spread operator (…) allows an iterable (like a string) to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected.
JavaScript Code:
function reverseString(str) {
return [...str].reverse().join("");
}
console.log(reverseString("Hello World"));
Output:
dlroW olleH
Explanation:
- Spread Operator (…str): The spread operator is used to expand the string str into an array of characters. Each character of the string is treated as a separate element in the array. This step is crucial because the reverse() method works on arrays, not directly on strings.
- .reverse(): This method reverses the array in place. The first array element becomes the last, and the last array element becomes the first. It’s important to note that strings in JavaScript are immutable, so we need to work with an array to perform the reversal.
- .join(“”): After reversing the array, this method joins all elements of the array back into a string. The argument “” (empty string) tells the method to join the array elements without adding any additional characters between them.
This approach using the spread operator is not only concise but also leverages modern JavaScript features (ES6) to simplify string and array manipulation. It’s a great example of how newer language features can make code more readable and expressive, especially for tasks like finding reverse of a string in JavaScript.
Also Read: How to Print Multiplication Table in JavaScript? (using for, while, recursion, html)
Interview Questions for You to Prepare for Jobs
Reverse a String in JavaScript Using While Loop
Reverse of a string in JavaScript using a while loop involves manually iterating over the string from the end to the beginning and constructing a new string by appending each character.
This method gives a deeper understanding of loops and string manipulation without relying on built-in array methods. It’s a more basic approach that showcases fundamental programming concepts.
JavaScript Code:
function reverseString(str) {
let reversed = "";
let index = str.length - 1;
while (index >= 0) {
reversed += str[index];
index--;
}
return reversed;
}
console.log(reverseString("Welcome to WsCube Tech"));
Output:
hceT ebuCsW ot emocleW
Explanation:
- Initialization: Start by creating an empty string reversed that will eventually hold the reversed string. Also, initialize index to the last character of the string (str.length – 1).
- While Loop: The loop continues as long as index is greater than or equal to 0. This condition ensures that each character of the string is visited, starting from the last character and moving to the first.
- Building the Reversed String: In each iteration, the character at the current index is concatenated to reversed. This gradually builds the reversed string as the loop progresses.
- Decrementing index: After each iteration, index is decremented by 1, moving the focus one character to the left in the original string.
- Return: Once the loop completes (when index < 0, meaning every character has been processed), the function returns the reversed string.
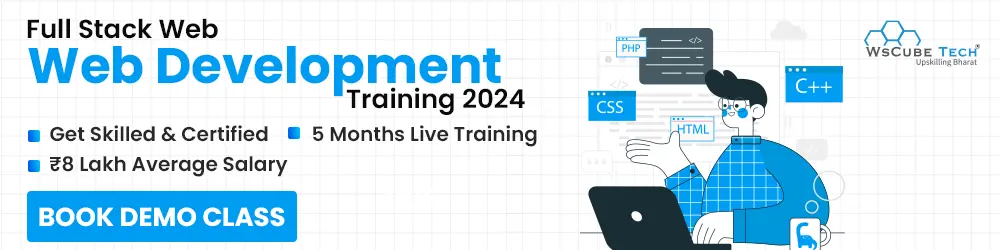
Reverse String in JavaScript Using Array
To reverse a string in JavaScript using Array.from() and the reverse() method, you essentially convert the string into an array of characters, reverse the array, and then join the characters back into a string.
This approach uses the Array.from() method to create an array from a string, which is particularly useful for handling strings with multi-byte characters or emojis correctly.
JavaScript Code:
function reverseString(str) {
// Convert the string to an array of characters, reverse it, and then join it back to a string
return Array.from(str).reverse().join('');
}
console.log(reverseString("Hello World")); // Output: "dlroW olleH"
console.log(reverseString("👋🌍"));
Output:
dlroW olleH
🌍👋
Explanation:
- Array.from(str): Converts the string str into an array of characters. Unlike str.split(”), Array.from() correctly handles characters represented by surrogate pairs (e.g., many emojis, certain special characters), ensuring they are not split into separate array elements. This is particularly important for strings containing such characters, as misinterpreting them could result in incorrect behavior or data loss.
- .reverse(): This method reverses the array of characters in place. The first array element becomes the last, and the last array element becomes the first.
- .join(”): After reversing the array, this method joins all elements of the array back into a string. The argument ” (empty string) tells the method to join the array elements without adding any additional characters between them.
Using Array.from() for string reversal ensures better handling of Unicode characters, making this method more robust and reliable for a wider range of strings, including those with complex characters.
Also Read: Find Factorial in JavaScript (3 Programs & Code)
How to Reverse a Number in JavaScript?
Now, finally, we are going to write a program to reverse a number in JavaScript.
JavaScript Code:
function reverseNumber(num) {
return parseFloat(
num
.toString() // Convert the number to a string
.split('') // Split the string into an array of characters
.reverse() // Reverse the array
.join('') // Join the array back into a string
) * Math.sign(num); // Convert the string back to a number and retain the original sign
}
console.log(reverseNumber(12345));
console.log(reverseNumber(-67890));
Output:
54321
-09876
Explanation:
- num.toString(): This method converts the number num to a string. This is necessary because the split, reverse, and join methods are intended for strings, not numbers.
- split(”): Splits the string representation of the number into an array of individual characters (digits).
- reverse(): Reverses the array of characters in place, so the last digit becomes the first, and so on.
- join(”): Joins the reversed array of characters back into a string.
- parseFloat(): Converts the string back into a floating-point number. This is used instead of parseInt to handle cases where the reversed number might start with 0, and also because it can handle both integer and decimal numbers.
- Math.sign(num): Multiplies the result by the sign of the original number to retain whether it was positive or negative. Math.sign(num) returns 1 for positive numbers, -1 for negative numbers, and 0 for zero, effectively keeping the sign of the original number intact.
Role of Using JavaScript Reverse String Program
The role of using the JavaScript program to reverse a string extends beyond the simple act of flipping a sequence of characters. This exercise serves multiple educational and practical purposes in programming and web development contexts:
1. Understanding String Manipulation
- Fundamental Skill: String manipulation is a fundamental skill in programming. Learning to reverse a string helps beginners understand how strings are treated in JavaScript, including concepts like immutability and methods for string manipulation.
- Broad Applicability: The skills learned are applicable to a wide range of programming tasks, such as formatting user input, generating slugs for URLs, or creating palindromes.
2. Grasping JavaScript Methods and Properties
- Method Usage: Reversing a string in JavaScript includes methods like split(), reverse(), and join(). Through this exercise, learners become familiar with these and other array and string methods, understanding their syntax, return values, and side effects.
- Property Access: It introduces accessing properties of strings and arrays, such as length, which is crucial for navigating through and manipulating these data structures.
3. Introduction to Loops and Conditional Statements
- Looping Constructs: For more manual approaches to reversing a string (such as using a for loop), students learn about looping constructs, iterating over arrays or strings, and building logic incrementally.
- Conditionals: In more complex variations, conditional statements might be used to handle special cases, teaching beginners how to make decisions within their code.
4. Familiarity with ES6+ Features
- Spread Operator: The task can be approached using modern JavaScript features like the spread operator, introducing learners to concise and powerful ES6+ syntax that makes code more readable and efficient.
- Arrow Functions: Combined with array methods, this task can also teach the use of arrow functions for callbacks, a staple in modern JavaScript development.
5. Problem-Solving Skills
- Algorithmic Thinking: Even a simple task like reversing a string can enhance algorithmic thinking, encouraging learners to break down problems into smaller parts and solve them systematically.
- Optimization: As learners explore different methods to reverse a string, they start to consider factors like performance and efficiency, important aspects of writing high-quality code.
6. Real-world Applications
- Data Transformation: In web development, transforming user input or data from APIs is common. Understanding how to manipulate strings is directly applicable to formatting or altering this data for display or processing.
- UI Effects: Certain UI effects, such as animations involving text, might require manipulation of strings to achieve desired visual outcomes.
7. Foundation for Advanced Topics
- Recursion: Some solutions to the string reversal problem may introduce recursion, setting the stage for understanding this important concept in computer science.
- Character Encoding: It can lead to discussions about character encoding and handling multi-byte characters or emojis, relevant for internationalization and working with diverse datasets.
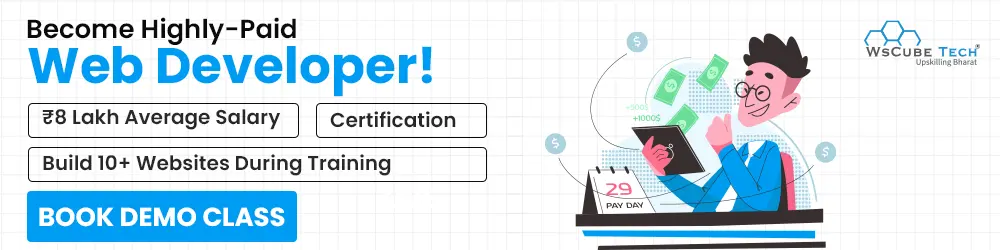
Also Read: How to Become Web Developer in 2024? Beginners Guide
Importance of Choosing the Right Method for Reverse String in JS
Choosing the right method to solve a programming task, such as reversing a string or a number in JavaScript, is crucial for several reasons, including performance, readability, maintainability, and resource efficiency:
1. Performance
- Efficiency: Different methods have varying computational complexities. For example, using built-in methods (split, reverse, join) for reversing a string is concise and fast for small to moderately sized strings. However, for very large strings or in performance-critical applications, this approach might not be the most efficient due to the creation of intermediate arrays and the overhead of multiple function calls.
- Big O Notation: Understanding the time complexity (e.g., O(n)) of an operation helps in predicting how the execution time will scale with input size. A method that is O(n) might be preferable over an O(n²) method for large inputs.
2. Readability and Maintainability
- Code Clarity: Choosing methods that make the code more readable and understandable to other developers is essential, especially in collaborative environments. While a recursive method might be elegant, a simple loop could be more straightforward for others (or even your future self) to understand and maintain.
- Convention and Best Practices: Adhering to common conventions and best practices in the community can also guide the choice of method. If a particular pattern or method is widely adopted, it may be more accessible to other developers.
3. Resource Efficiency
- Memory Usage: Some methods may require more memory than others. For instance, methods that involve creating additional data structures (like arrays in the split-reverse-join method) might use more memory than an in-place reversal using a loop, which could be significant in memory-constrained environments.
- CPU Time: The amount of CPU time required can vary significantly between methods. In a browser environment, a method that minimizes CPU usage might provide a smoother user experience by not blocking the main thread.
4. Contextual Considerations
- Environment: The execution environment (e.g., server-side Node.js vs. client-side JavaScript in a web browser) can influence the choice of method. Server-side environments might prioritize raw performance, while client-side code might focus on minimizing impact on user experience.
- Data Size: For small datasets, the choice of method might have a negligible impact on performance, making readability a higher priority. For large datasets, however, performance considerations might dictate the choice.
- Functionality vs. Optimization: Early in development, choosing more straightforward methods can expedite feature development and debugging. As the application matures, optimizing critical paths for performance becomes more important.
Free Courses for You
FAQs Related to JavaScript Reverse String
The simplest way is to use the combination of split(), reverse(), and join() methods. This is an in-built approach that splits the string into an array, reverses the array, and then joins it back into a string.
Due to strings in JavaScript being immutable (they cannot be changed after they are created), it’s not possible to reverse a string in-place in the same way you might reverse an array. Any operation that appears to modify a string will actually return a new string.
Using the spread operator to convert a string into an array of characters is similar to using split(“”) but can be more succinct and clearer to read. Additionally, the spread operator handles surrogate pairs (for Unicode characters represented by two code units) more reliably.
Recursion is a powerful and elegant solution, but it’s not always the most efficient, especially for very long strings. Recursive methods can lead to a stack overflow error if the string is too long or if the maximum call stack size is exceeded.
The performance can vary based on the JavaScript engine, the string’s length, and the specific operation’s context. For short strings, the difference is negligible, but for very large strings, methods that minimize intermediate steps (like avoiding creating additional arrays) may perform better.
You can reverse a string without built-in methods by manually iterating over the string with a loop, collecting characters in reverse order. This method requires more code but can be a useful exercise in understanding loops and string manipulation.
Yes, characters that are represented by surrogate pairs in Unicode (such as many emoji and some language characters) may not be handled correctly by simple reversal methods. These characters take up two positions in the string, and reversing the string without accounting for these pairs can result in invalid characters.
The reversal process itself should not modify the encoding or characters, but care should be taken with characters represented by surrogate pairs, as mentioned. Ensuring that the reversal method correctly handles these cases is essential for preserving the original content accurately.
To correctly reverse a string containing emojis or other multibyte characters, you can use the spread operator to ensure that surrogate pairs are treated as single characters, or use a library that is aware of Unicode and can properly handle these cases.
Reversing a string can be useful in various scenarios, such as creating palindromes, generating reverse domains for DNS lookups, text animation effects, or simply as a programming exercise to learn string manipulation techniques.
Wrapping Up:
Mastering how to reverse a string in JavaScript opens the door to understanding more complex programming concepts and enhancing your problem-solving skills. While we’ve explored various methods to achieve this, the journey of learning and improvement doesn’t stop here.
For those looking to dive deeper into JavaScript or elevate their career in web development, enrolling in a live online JavaScript course or a comprehensive full-stack developer course can be a game-changer.
These courses not only offer personalized guidance and real-world projects but also equip you with the skills needed to excel in the ever-evolving tech landscape.
Read more blogs: