In our series of JavaScript programming examples, today, we are going to learn how to build a simple calculator using JS.
So, what do we mean by a simple calculator? Imagine the basic calculator app on your phone or computer. It does straightforward tasks like addition, subtraction, multiplication, and division. That’s exactly what we’ll be creating.
But instead of just using it, you’ll learn how to make a simple calculator in JavaScript from scratch.
Why does this matter?
Building a simple calculator might seem basic, but it’s a stepping stone to mastering web development. It helps you understand the fundamentals of JavaScript, HTML, and CSS. More importantly, it teaches you how to make something interactive that works on a web page, which is a crucial web development skill.
And what better way to practice than by building real, functional projects? This is not just about learning to code; it’s about thinking like a developer and solving problems like one.
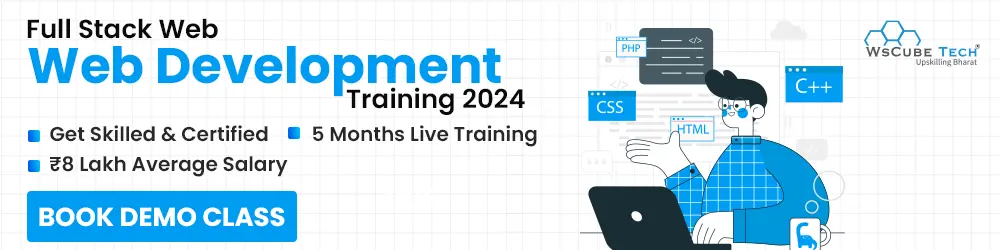
For those who find this exciting and wish to take a step further, consider enrolling in the live online full-stack developer course by WsCube Tech. It takes you beyond the basics, covering both front-end and back-end development, and prepare you for a career in building complete websites and web applications.
Simple Calculator Using JavaScript, HTML, and CSS
Let’s learn how to build a simple calculator using JavaScript, HTML, and CSS.
Code:
<!-- Program to build Simple Calculator in JavaScript using HTML and CSS. -->
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Calculator </title>
<style>
h1 {
text-align: center;
padding: 23px;
background-color: purple;
color: white;
}
#clear{
width: 270px;
border: 3px solid black;
border-radius: 3px;
padding: 20px;
background-color: #000000;
color: white;
}
.formstyle {
width: 300px;
height: 530px;
margin: auto;
border: 3px solid purple;
border-radius: 5px;
padding: 20px;
}
input {
width: 20px;
background-color: #8A2BE2;
color: white;
border: 3px solid black;
border-radius: 5px;
padding: 26px;
margin: 5px;
font-size: 15px;
}
#calc
width: 250px;
border: 5px solid black;
border-radius: 3px;
padding: 20px;
margin: auto;
}
</style>
</head>
<body>
<h1> JavaScript Simple Calculator by WsCube Tech </h1>
<div class="formstyle">
<form name="form1">
<!-- This input box shows the button pressed by the user in calculator. -->
<input id="calc" type="text" name="answer"> <br> <br>
<!-- Display the calculator button on the screen. -->
<!-- onclick() function display the number pressed by the user. -->
<input type="button" value="1" onclick="form1.answer.value += '1' ">
<input type="button" value="2" onclick="form1.answer.value += '2' ">
<input type="button" value="3" onclick="form1.answer.value += '3' ">
<input type="button" value="+" onclick="form1.answer.value += '+' ">
<br> <br>
<input type="button" value="4" onclick="form1.answer.value += '4' ">
<input type="button" value="5" onclick="form1.answer.value += '5' ">
<input type="button" value="6" onclick="form1.answer.value += '6' ">
<input type="button" value="-" onclick="form1.answer.value += '-' ">
<br> <br>
<input type="button" value="7" onclick="form1.answer.value += '7' ">
<input type="button" value="8" onclick="form1.answer.value += '8' ">
<input type="button" value="9" onclick="form1.answer.value += '9' ">
<input type="button" value="*" onclick="form1.answer.value += '*' ">
<br> <br>
<input type="button" value="/" onclick="form1.answer.value += '/' ">
<input type="button" value="0" onclick="form1.answer.value += '0' ">
<input type="button" value="." onclick="form1.answer.value += '.' ">
<!-- When we click on the '=' button, the onclick() shows the sum results on the calculator screen. -->
<input type="button" value="=" onclick="form1.answer.value = eval(form1.answer.value) ">
<br>
<!-- Display the Cancel button and erase all data entered by the user. -->
<input type="button" value="Clear All" onclick="form1.answer.value = '' " id="clear" >
<br>
</form>
</div>
</body>
</html>
Output:
On running the code, you will see the following output:
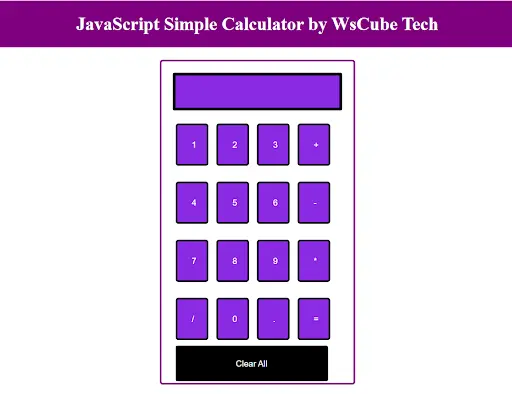
It is an interactive JavaScript calculator. You can use it by pressing the buttons and easily perform calculations.
Explanation:
HTML Structure
- <h1> Tag: Displays the calculator’s title at the top of the page with a centered alignment.
- <div class=”formstyle”>: Encapsulates the calculator’s form, providing a styled container.
- <form name=”form1″>: Defines the form that holds the calculator’s input and buttons.
Input and Buttons
- <input id=”calc” type=”text” name=”answer”>: A text input field that displays the user’s input and the calculation result.
- Value Buttons (1, 2, 3, etc.): These buttons have an onclick attribute that appends their value to the text input when clicked.
- Operation Buttons (+, -, *, /): Similar to value buttons but for arithmetic operations. They append their respective operation symbol to the input field.
- Equals Button (=): Evaluates the expression in the input field using the eval function and displays the result.
- Clear Button (Clear All): Clears the input field, allowing the user to start a new calculation.
CSS Styling
- h1: Styles the header with a purple background and white text.
- #clear: Styles the clear button with a black background and border.
- .formstyle: Styles the calculator’s form container with a border and padding.
- input: Applies styling to all input elements, including buttons, with a focus on the purple theme for the calculator buttons and a black border.
- #calc: Specifically styles the main calculator display input to differentiate it from other buttons.
Upskill Yourself With Live Training
Recommended Courses for You | Book Live Class for FREE! |
Full Stack Web Development Course | Book Now |
WordPress Course | Book Now |
Front-End Development Course | Book Now |
JavaScript Program for Calculator (Without HTML & CSS)
Below is a straightforward JavaScript program to make a simple calculator that performs basic arithmetic operations based on user input.
This example uses prompt to get input from the user and alert to show the result. If you’re running this in an environment that doesn’t support prompt or alert (like some online compilers), you might need to use console.log or another method to input and output data.
JavaScript Code:
// Function to ask for the operation
function getOperation() {
const operation = prompt("Choose an operation: +, -, *, /");
return operation;
}
// Function to ask for numbers
function getNumbers() {
const num1 = parseFloat(prompt("Enter first number:"));
const num2 = parseFloat(prompt("Enter second number:"));
return { num1, num2 };
}
// Perform the calculation based on the operation
function calculate(operation, num1, num2) {
switch (operation) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
// Check for division by zero
if (num2 === 0) {
return "Cannot divide by zero";
}
return num1 / num2;
default:
return "Invalid operation";
}
}
// Main function to run the calculator
function runCalculator() {
const operation = getOperation();
const { num1, num2 } = getNumbers();
const result = calculate(operation, num1, num2);
alert(`Result: ${result}`);
}
// Run the calculator
runCalculator();
Output:
Choose an operation: +, -, *, /
+
Enter first number:10
Enter second number:43
Result: 53
Explanation:
getOperation Function:
- It prompts the user to choose an arithmetic operation from +, -, *, or /.
- The chosen operation is then returned for use in the calculation.
getNumbers Function:
- This function prompts the user twice, asking for the first and second numbers to be used in the calculation.
- It uses parseFloat to convert the input from strings to floating-point numbers, ensuring that arithmetic operations can be performed accurately.
- The numbers are returned as properties of an object: { num1, num2 }.
calculate Function:
- Given the operation (as a string) and two numbers, this function performs the appropriate arithmetic operation.
- It uses a switch statement to determine which operation to execute based on the input.
- For division, it includes a check to ensure that division by zero is handled gracefully, returning a specific message if attempted.
- If the operation is not recognized, it returns a message indicating an invalid operation.
runCalculator Function:
- This is the main function that orchestrates the calculator’s operation.
- It first calls getOperation to obtain the arithmetic operation from the user.
- Then, it calls getNumbers to get the two numbers for the calculation.
- With these inputs, it calls calculate to perform the operation on the numbers.
- Finally, it displays the result of the calculation using alert.
Execution:
- The runCalculator function is called to start the calculator. This initiates the sequence of prompts for the user and ultimately displays the result.
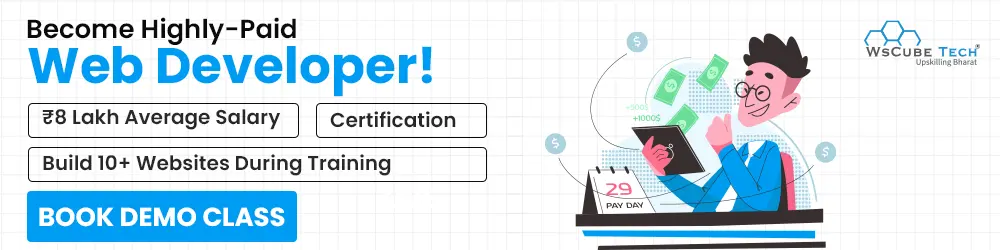
Simple JavaScript Calculator Code Using Switch
Here, we will write a JavaScript program for calculator using switch:
// Function to perform the calculation based on the operation
function calculate(operation, num1, num2) {
let result;
switch (operation) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 === 0) {
console.log("Error: Division by zero is not allowed.");
return;
}
result = num1 / num2;
break;
default:
console.log("Error: Invalid operation.");
return;
}
console.log(`${num1} ${operation} ${num2} = ${result}`);
}
// Simulated input using prompt (replace with actual prompt in a supportive environment)
let operation = prompt("Enter operator: "); // '+' for addition
let num1 = parseFloat(prompt("Enter first number: ")); // e.g., 4
let num2 = parseFloat(prompt("Enter second number: ")); // e.g., 5
// Perform the calculation
calculate(operation, num1, num2);
Output:
Enter operator: +
Enter first number: 90
Enter second number: 10
90 + 10 = 100
Explanation:
Function: calculate(operation, num1, num2)
- Parameters: It takes an operation (a string representing the arithmetic operation) and two numbers (num1 and num2) as arguments.
- Operation Handling: Uses a switch statement to execute different code blocks based on the operation provided (+, -, *, /).
- Result Output: For valid operations, it calculates the result and logs the operation along with the result to the console (e.g., “4 + 5 = 9”).
- Error Handling: If an invalid operation is entered, it logs an error message indicating the operation is not supported.
Input Simulation
- The code simulates obtaining user input through prompt for the operation type (+, -, *, /) and two numeric values. These prompts are placed outside the calculate function for demonstration purposes.
- parseFloat is used to convert the input strings to floating-point numbers, ensuring arithmetic operations are performed correctly.
Execution Flow
- The user is prompted to enter an operation and two numbers.
- These inputs are passed to the calculate function.
- The function performs the specified operation on the numbers and logs the result to the console.
Interview Questions for You to Prepare for Jobs
Dynamic Calculator Using JavaScript, HTML, and CSS
Now, we will create a dynamic and simple calculator using JavaScript, HTML, and CSS.
Code
<!DOCTYPE html>
<html>
<head>
<title>Calculator Program in JavaScript</title>
<script>
// Use insert() function to insert the number in textview.
function insert(num) {
document.form1.textview.value = document.form1.textview.value + num;
}
// Use equal() function to return the result based on passed values.
function equal() {
var exp = document.form1.textview.value;
if(exp) {
document.form1.textview.value = eval(exp);
}
}
/* Here, we create a backspace() function to remove the number at the end of the numeric series in textview. */
function backspace() {
var exp = document.form1.textview.value;
document.form1.textview.value = exp.substring(0, exp.length - 1); /* remove the element from total length - 1 */
}
</script>
<style>
/* Create the Outer layout of the Calculator. */
.formstyle {
width: 300px;
height: 330px;
margin: 20px auto;
border: 3px solid purple;
border-radius: 5px;
padding: 20px;
text-align: center;
background-color: #6a0dad;
}
/* Display top horizontal bar that contain some information. */
h1 {
text-align: center;
padding: 23px;
background-color: purple;
color: white;
}
input:hover {
background-color: #8A2BE2;
}
* {
margin: 0;
padding: 0;
}
/* It is used to create the layout for calculator button. */
.btn {
width: 50px;
height: 50px;
font-size: 25px;
margin: 2px;
cursor: pointer;
background-color: black;
color: white;
}
/* It is used to display the numbers, operations and results. */
.textview {
width: 223px;
margin: 5px;
font-size: 25px;
padding: 5px;
background-color: #D8BFD8;
}
</style>
</head>
<body>
<h1> Dynamic JavaScript Calculator by WsCube Tech </h1>
<div class="formstyle">
<form name="form1">
<input class="textview" name="textview">
</form>
<center>
<table>
<tr>
<td> <input class="btn" type="button" value="C" onclick="form1.textview.value = '' " > </td>
<td> <input class="btn" type="button" value="B" onclick="backspace()" > </td>
<td> <input class="btn" type="button" value="/" onclick="insert('/')" > </td>
<td> <input class="btn" type="button" value="x" onclick="insert('*')" > </td>
</tr>
<tr>
<td> <input class="btn" type="button" value="7" onclick="insert(7)" > </td>
<td> <input class="btn" type="button" value="8" onclick="insert(8)" > </td>
<td> <input class="btn" type="button" value="9" onclick="insert(9)" > </td>
<td> <input class="btn" type="button" value="-" onclick="insert('-')" > </td>
</tr>
<tr>
<td> <input class="btn" type="button" value="4" onclick="insert(4)" > </td>
<td> <input class="btn" type="button" value="5" onclick="insert(5)" > </td>
<td> <input class="btn" type="button" value="6" onclick="insert(6)" > </td>
<td> <input class="btn" type="button" value="+" onclick="insert('+')" > </td>
</tr>
<tr>
<td> <input class="btn" type="button" value="1" onclick="insert(1)" > </td>
<td> <input class="btn" type="button" value="2" onclick="insert(2)" > </td>
<td> <input class="btn" type="button" value="3" onclick="insert(3)" > </td>
<td rowspan="5"> <input class="btn" style="height: 110px" type="button" value="=" onclick="equal()"> </td>
</tr>
<tr>
<td colspan="2"> <input class="btn" style="width: 106px" type="button" value="0" onclick="insert(0)" > </td>
<td> <input class="btn" type="button" value="." onclick="insert('.')"> </td>
</tr>
</table>
</center>
</div>
</body>
</html>
Output:
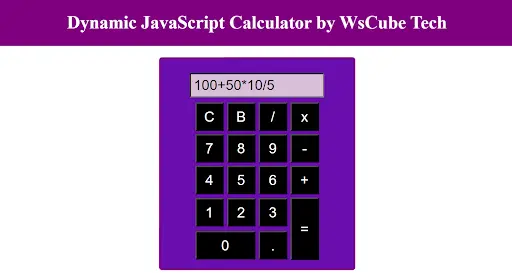
Explanation:
This code snippet creates a basic calculator using JavaScript, HTML, and CSS.
HTML Structure
- <h1> Tag: Displays the title of the calculator in a header, centered at the top.
- <div class=”formstyle”>: Encapsulates the calculator’s interface, including the input field and buttons. This division is styled to look like a calculator.
- <form name=”form1″>: Contains the input element where calculations are displayed and where the result is shown.
- <input class=”textview” name=”textview”>: The text input field where numbers and results are displayed.
- Buttons (0-9, +, -, *, /, C, B, =): Each button is represented by an <input type=”button”> with an onclick event to perform its specific function, such as adding a digit to the display or performing a calculation.
CSS Styling
- .formstyle: Styles the calculator’s container, including its size, background color (grey, changed to purple in the modified version), and border.
- h1: Styles the header with a background color (skyblue, changed to purple in the modified version) and text color.
- .btn: Applies styles to the calculator buttons, including size, background color (red, changed to black in the modified version), and hover effect.
- .textview: Styles the display input where numbers and results are shown, with a background color (lightgreen, adjusted in the modified version for contrast).
JavaScript Functions
- insert(num): Appends the pressed button’s number or symbol to the display input.
- equal(): Evaluates the expression shown in the display input using the eval function and shows the result. It handles basic arithmetic operations entered by the user.
- backspace(): Removes the last character from the display input, allowing the user to correct mistakes.
Functionality
- The calculator allows users to input numbers and select operations to perform basic calculations.
- The C button clears the display, the B button deletes the last entered character, and the = button computes the expression.
This code is a great starting point for learners to understand how to create interactive web elements with HTML, style them with CSS, and make them functional with JavaScript. It shows the integration of form inputs, event handling, and basic JavaScript operations in a practical, user-friendly application.
Also read: How to Reverse String in JavaScript? 7 Ways & Programs
Role of Using JavaScript Calculator
The role of JavaScript code for a calculator in web development, especially for beginners, serves several key purposes that extend beyond just the creation of a simple application. It encompasses learning fundamental concepts, practicing coding skills, and understanding the intricacies of web development. Let’s explore these aspects in more detail:
1. Understanding Basic Programming Concepts
Building a JavaScript calculator introduces you to basic programming concepts that are essential for any coding journey.
These include:
- Variables and Data Types: Learn how to store and manipulate data, using numbers and strings, which are crucial for operations and displaying results.
- Functions: Understand how to create reusable blocks of code that perform specific tasks, such as adding or subtracting numbers.
- Control Structures: Get to grips with if-else statements and loops, which can help in decision-making processes within your code.
2. Learning JavaScript Syntax and Best Practices
JavaScript is a leading web development language, and mastering its syntax is key to developing dynamic websites.
By building a calculator in JavaScript:
- You’ll write real JavaScript code, helping you understand how to structure your code effectively.
- You’ll learn about best practices in coding, such as naming conventions, commenting, and organizing your code, making it readable and maintainable.
3. Enhancing Problem-Solving Skills
Developing a JavaScript calculator requires logical thinking and problem-solving skills. You’ll need to figure out how to:
- Capture user input correctly.
- Perform calculations and handle potential errors, such as division by zero.
- Ensure the user interface is intuitive and the calculator is user-friendly.
4. Introduction to Debugging
As with any coding project, you’re bound to encounter bugs. A JavaScript calculator project forces you to practice debugging, teaching you how to:
- Use browser developer tools to inspect code and monitor variables.
- Understand error messages and learn how to fix issues effectively.
- Test your application to ensure it works as expected under different scenarios.
5. Foundation for More Complex Projects
Finally, a calculator in JavaScript might seem simple, but it lays the groundwork for tackling more complex projects. It’s a practical way to apply what you’ve learned and serves as a confidence booster.
After completing it, you’ll be better prepared to explore more advanced topics like web APIs, frameworks like React or Angular, and even server-side programming.
Also read: Program for Armstrong Number in JavaScript (5 Ways)
Mistakes to Avoid in JavaScript Calculator Programs
Writing a JavaScript calculator script can be a rewarding project for beginners, but there are common mistakes that you should be aware of to ensure your calculator works correctly and efficiently:
1. Misusing the eval() Function
- Issue: The eval() function evaluates a string as JavaScript code. While it might seem convenient for calculating expressions entered by the user, it poses security risks and can lead to inefficient code.
- Solution: Use a safer alternative, such as parsing the expressions manually or using a library designed for mathematical evaluations.
2. Not Handling Division by Zero
- Issue: Division by zero in mathematics is undefined. Failing to handle this in your calculator can lead to errors or misleading results.
- Solution: Check if the denominator is zero before performing division and provide a meaningful message or handling mechanism.
3. Inaccurate Floating Point Arithmetic
- Issue: JavaScript has precision issues with floating-point arithmetic due to how numbers are stored in memory. This can lead to inaccuracies in calculations involving decimal numbers.
- Solution: Use the toFixed() method to limit the number of decimal places, or consider using a library that can handle arbitrary precision arithmetic.
4. Failing to Sanitize Input
- Issue: Accepting raw input without validation or sanitization can lead to errors or unexpected behavior, especially if you’re using eval() or dynamically generating code based on user input.
- Solution: Always validate and sanitize user inputs to ensure they meet the expected format and range. Avoid using user input directly in functions like eval().
5. Ignoring User Interface and Experience
- Issue: Overlooking the importance of a clear and intuitive user interface can lead to a calculator that’s difficult to use.
- Solution: Provide clear feedback for actions (e.g., button clicks), and ensure the user can easily enter expressions, clear input, or correct mistakes.
6. Overcomplicating the Solution
- Issue: Beginners often try to implement advanced features before mastering the basics, leading to complicated and buggy code.
- Solution: Start simple. Ensure your calculator can handle basic arithmetic before adding more complex functionalities like handling parentheses or scientific operations.
7. Not Accounting for Operator Precedence
- Issue: If your calculator evaluates expressions from left to right without considering operator precedence (e.g., multiplication before addition), it can yield incorrect results.
- Solution: Implement an algorithm that respects operator precedence, such as the shunting yard algorithm, or use existing libraries to parse expressions.
8. Poor Error Handling
- Issue: Not properly handling errors or invalid inputs can make your calculator frustrating to use.
- Solution: Implement clear error messages and feedback for invalid operations or inputs. Try to catch and handle potential errors gracefully.
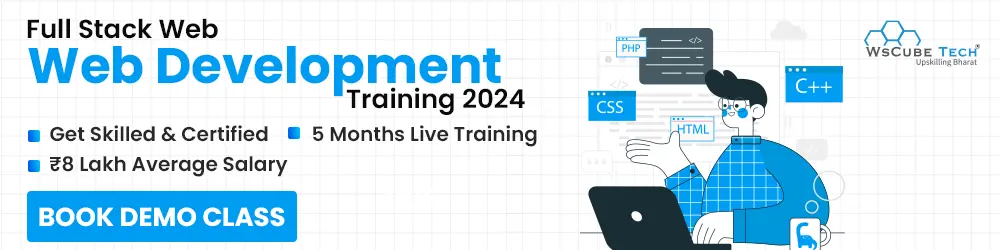
FAQs About JavaScript Calculator
A JavaScript calculator is a web-based application that allows users to perform arithmetic operations directly within a web browser. It uses HTML for the structure, CSS for styling, and JavaScript for functionality.
A JavaScript calculator captures user inputs through clickable buttons or keyboard inputs, processes the arithmetic operations in JavaScript, and then displays the results on the web page. It relies on event listeners to detect user actions and functions to perform calculations.
Yes, JavaScript can be used to create calculators for complex mathematical operations, including trigonometric functions, logarithms, and power calculations. For advanced mathematical functions, you might consider using the Math object in JavaScript or external libraries like math.js for more complex operations.
JavaScript sometimes produces long decimals in calculations due to floating-point arithmetic issues common in many programming languages. To address this, you can use the toFixed() method to limit the number of digits after the decimal point, though be aware that toFixed() returns a string.
Avoid using the eval() function, as it can execute arbitrary code and pose a security risk. Instead, parse the input manually or use secure libraries to evaluate expressions. Always validate and sanitize user input, even if not using eval().
Yes, you can create a scientific calculator using JavaScript. This involves programming additional functionalities such as handling parentheses, recognizing mathematical constants, and implementing various scientific operations. Libraries like math.js can simplify this process.
To correctly handle operator precedence without using eval(), you may need to implement a parsing algorithm, such as the shunting yard algorithm, which converts the infix notation (standard mathematical notation) to postfix notation (RPN – Reverse Polish Notation) before evaluation.
Yes, you can enhance your calculator to respond to keyboard inputs by adding event listeners for the keydown or keypress events in JavaScript. Map each key to its corresponding button or function in the calculator.
Division by zero is mathematically undefined. Calculators handle this scenario differently to avoid errors or the program crashing. Typically, a message is displayed, or the operation is ignored when a division by zero attempt is detected.
Testing can be done manually by performing operations and verifying the results. For automated testing, you can use JavaScript testing frameworks like Jest or Mocha to write test cases that cover various calculation scenarios, including edge cases and operator precedence.
Create a function in JavaScript that sets the value displayed in your calculator’s screen (usually an input field or a div) back to zero or an empty string. Attach this function to a ‘Clear’ button using an event listener.
Yes, and it’s recommended for security reasons. You can parse the input string and calculate the result using custom functions for each operation. This approach requires more code but avoids the security risks associated with eval().
Handling operator precedence without eval() involves parsing the input to identify numbers and operators, then applying the operations in the correct order. Implementing a parsing algorithm, such as the shunting-yard algorithm, helps manage precedence effectively.
Organize your code by separating concerns: use HTML for structure, CSS for styling, and JavaScript for functionality. In JavaScript, define functions for each operation, input handling, and the calculation process. Consider using object-oriented programming (OOP) principles to encapsulate calculator functionality.
Ensure that after every operation, the result is correctly set to the display element. Common issues include not updating the correct HTML element or having a mistake in the logic that generates the result.
Consider implementing scientific functions (sin, cos, tan, logarithms), memory storage (MC, MR, M+, M-), and support for complex expressions (handling parentheses and operator precedence).
Free Courses for You
Course Name | Course Name |
Django Course | Affiliate Marketing Course |
Semrush Course | Video Editing Course |
Blogging Course | AngularJS Course |
Shopify Course | Photoshop Course |
Wrapping Up:
Creating a JavaScript calculator is an excellent starting point for anyone interested in web development, offering a hands-on approach to learning key programming concepts.
However, to truly excel in this field and broaden your career opportunities, consider enrolling in a live online JavaScript course or a full stack developer course. These comprehensive programs will not only deepen your understanding of JavaScript but also equip you with the necessary skills to build complex applications, making you a highly sought-after professional in the tech industry.
Upskill today and take a significant step towards building a successful career in web development.
Read more blogs: